Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial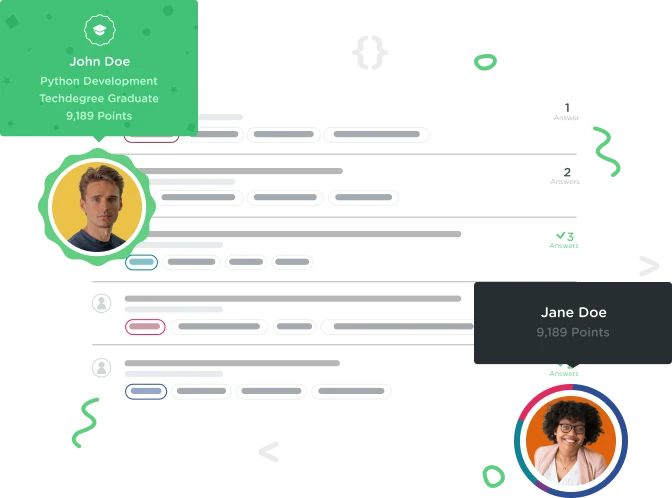
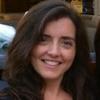
dotz
6,733 PointsReally stumped. What if the user provides a number lower than the first number chosen?
I have watched the videos several times. Did I miss somewhere that the user is expected to provide a number higher than his previously given number?
3 Answers

LaVaughn Haynes
12,397 PointsI think that in order to keep the example simple we just assume that the user will enter a larger number. Of course, even if they entered a smaller number the script would still work. For example if you typed in 1000 for the starting number and 1 for the top number you might get:
Math.floor(Math.random() * (1000 - 1 + 1)) + 1000;
// which could work out to -374 + 1000
// which would output "646 is a number between 1000 and 1"
You could always force the user to enter a higher second number if you want to. For example for the second number:
do{
secondNumber = prompt("Please enter a second number that is larger than the starting number");
}while(secondNumber <= firstNumber);
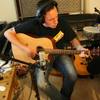
Benjamin Gooch
20,367 PointsSo I spent about an hour trying to figure this problem out (this is my first attempt at learning JavaScript) and I finally found a solution to this issue (though conditional statements aren't introduced until the next section) by digging around on MDN. I'm pretty happy with my resulting work!
//Prompt for user to enter a random number
//Number is converted to an Integer if it isn't already
var userInput1 = prompt('Please think of a number and type it below.');
userInput1 = parseInt(userInput1);
console.log(userInput1);
//Prompt for user to enter a second random number
//Number is converted to an Integer if it isn't already
var userInput2 = prompt('Thank you. Now please select a different number at random and type it below.');
userInput2 = parseInt(userInput2);
console.log(userInput2);
//Message displayed declaring a random number is to be selected by the computer
alert('Now I will pick a random number between the two numbers you have selected.');
console.log('Alert displayed');
//function to figure out the lower number
function lowerSelector() {
if (userInput1 < userInput2) {
lowerNumber = userInput1;}
else {
lowerNumber = userInput2;}
}
//Function to figure out the higher number
function higherSelector() {
if (userInput1 < userInput2) {
higherNumber = userInput2;}
else {
higherNumber = userInput1;}
}
//Running the Functions
lowerSelector();
document.write('Lower Number: ' + lowerNumber + '<br/>');
higherSelector();
document.write('Higher Number: ' + higherNumber + '<br/>');
//Generating the random number within range provided
var result = Math.floor(Math.random() * (higherNumber - lowerNumber + 1)) + lowerNumber;
document.write('Your random number between the specified values is: ' + result + '!');
alert('Your number is ' + result + '!');

Dave Switzer
2,581 PointsGood solution!!
You can also use a couple of other Math functions, min and max.
Here's what I did after the user entered the numbers:
var maxNum = Math.max(userNum, userNum2);
var minNum = Math.min(userNum, userNum2);
just thought I would throw another solution in there for you.
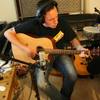
Benjamin Gooch
20,367 PointsGood solution, Dave. I'm still new to JavaScript (I only know what I've learned in this course so far) so I didn't know those functions existed!