Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial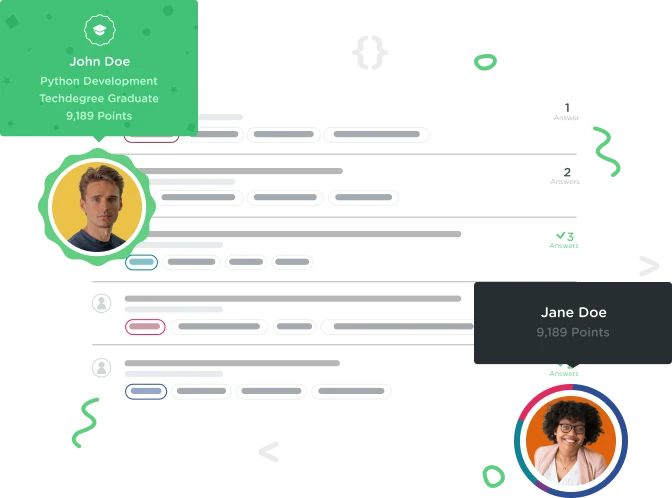

Andrew Chung
Courses Plus Student 4,094 PointsRebinding the checkbox change event handler
Check out the code snippet below and compare the last line between both functions. In terms of rebinding the checkbox change event's handler, both sets of code seem to work. If both sets of code below are valid, what are the performance implications (if any) between using one over the other?
//Original Code
var taskIncomplete = function () {
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
//Modified Code
var taskIncomplete = function () {
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
this.onchange = taskCompleted;
}
I'm basing this on my understanding of this project so far (and please correct me If I'm wrong on anything here - would really appreciate the feedback):
Each time the change event on any task's checkbox is triggered, we are calling an event handler. The event handler for checkboxes whose tasks are in the TODO list (i.e. incompleteTasksHolder) is taskCompleted, while the event handler for checkboxes whose tasks in the COMPLETED list (i.e. completedTasksHolder) is taskIncomplete. This allows us to move tasks from the TODO list to the COMPLETED list, and vice versa.
When our project files first load, the two for loops in our app.js file will bind event handlers to the click events for the Edit and Delete buttons, and bind either the taskCompleted or taskIncomplete event to each checkbox's change event, depending on which list the task is originally a part of.
When we move a task from one list to the other by clicking on the checkbox, we need to rebind the event handler on that checkbox's change event so that the task doesn't get stuck. For a task that is originally in the TODO list, its checkbox's change event will be bound to the taskCompleted event handler (i.e., when you click on a checkbox in the TODO list, it should move that checkbox and its task to the COMPLETED list). If we click on the checkbox again when it's in the COMPLETED list, it'll call the taskCompleted event and re-append itself to the COMPLETED list.
SO...that was a lot of text! But if what I've described is correct, then wouldn't it make more sense to use the Modified Code above? I can see why we're calling bindTaskEvents when we add a new task, since our JS file only binds event handlers to tasks that existed when our files originally loaded. However, it seems like we don't need to rebind the event handlers on the Edit and Delete buttons' events for already existing tasks, in which case calling bindTaskEvents seems a bit overkill.

sibal gesekki
3,484 Pointsandrew didnt think his lesson out properly smh

Alejandro Molina
3,997 PointsWow. This actually makes far more sense than what Andrew did. I am impressed.
Erik Nuber
20,629 PointsErik Nuber
20,629 PointsThis definitely makes more sense and, it works. Events happen so quickly on a computer that you can't tell the difference time-wise but, this functionality seems better than calling a function when the items are already bound.