Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial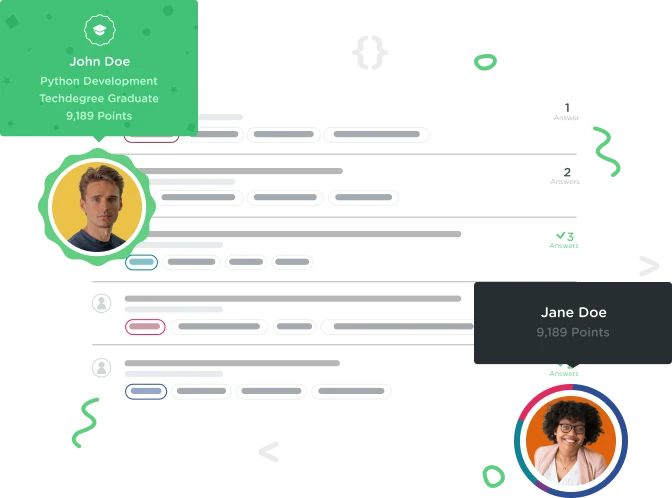
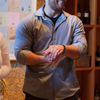
Jonathan Ankiewicz
17,901 PointsReceiving Form data from a POST method. Receiving multipart/form-data object.
I am using a node express application server.
I am trying to push and parse form data from a form built with angular.
The single page is built on my express server I am sending a POST method from the form to the route '/quote' and trying to grab the data. Because I am sending the data from the form and not as JSON. I am having trouble returning my results. I don't recall the courses covering different types of data.
Can someone help me with this or/and point me in the right direction to read documentation on finding a the correct resolve. I looked into npm multer node(bad idea, installed it and crashed my node, I had to uninstall and reinstall everything.
I only used angular for form validation. (trying to flex my new skills:Shout out to Full Stack Javascript Path)
<div class="row">
<div class="col-md-6 col-md-offset-3">
<h2 class="page-header">Register</h2>
<div ng-app="submitForm" ng-controller="mainCtrl">
<form method="post" action="/quote">
<div class="form-group">
<label>Name</label>
<input type="text" class="form-control" placeholder="Name" name="name" ng-model="frm.name" required>
</div>
<div class="form-group">
<label>Username</label>
<input type="text" class="form-control" placeholder="Username" name="username" ng-model="frm.username" required>
</div>
<div class="form-group">
<label>Email</label>
<input type="email" class="form-control" placeholder="Email" name="email" ng-model="frm.email" required>
<span class="errMessage" ng-show="frm.email.$dirty && frm.email.$error.required">Required</span>
<span class="errMessage" ng-show="frm.email.$dirty && frm.email.$error.email">Not an Email</span>
</div>
<div class="form-group">
<label>Phone Number</label>
<input type="Phone" class="form-control" placeholder="Phone" name="Phone" ng-model="frm.phone" required>
</div>
<div class="form-group">
<label>Other notes</label>
<textarea type="textarea" class="form-control" placeholder="Comment" name="comment" ng-model="frm.content"></textarea>
</div>
<button ng-disabled="frm.$invalid" type="submit" ng-click="sendEmail()" class="btn btn-default">Submit</button>
<pre>
{{ frm }}
{{ frm.email.$dirty }}
{{ frm.email.$error.required }}
</pre>
</form>
</div>
</div>
</div>
if (!databaseUri) {
console.log('DATABASE_URI not specified, falling back to localhost.');
}
var api = new ParseServer({
databaseURI: databaseUri || 'mongodb://localhost:27017/dev',
cloud: process.env.CLOUD_CODE_MAIN || __dirname + '/cloud/main.js',
appId: process.env.APP_ID || 'myAppId',
masterKey: process.env.MASTER_KEY || 'master', //Add your master key here. Keep it secret!
serverURL: process.env.SERVER_URL || 'http://localhost:1337/parse', // Don't forget to change to https if needed
liveQuery: {
classNames: ["Posts", "Comments"] // List of classes to support for query subscriptions
}
});
// Client-keys like the javascript key or the .NET key are not necessary with parse-server
// If you wish you require them, you can set them as options in the initialization above:
// javascriptKey, restAPIKey, dotNetKey, clientKey
var app = express();
// Json parser middleware
app.use(jsonParser());
app.use(function(req, res, next) {
if (req.body) {
console.log("The sky is");
} else {
console.log("Didn't work");
}
next();
});
// handlebars views
app.set('views', path.join(__dirname, 'views'));
app.engine('handlebars', exphbs({ defaultLayout: 'layout' }));
app.set('view engine', 'handlebars');
// Serve static assets from the /public folder
app.use('/public', express.static(path.join(__dirname, '/public')));
app.use('/quote', express.static(path.join(__dirname, '/quote')));
// Serve the Parse API on the /parse URL prefix
var mountPath = process.env.PARSE_MOUNT || '/parse';
app.use(mountPath, api);
// Parse Server plays nicely with the rest of your web routes
app.get('/', function(req, res) {
// res.status(200).send('I dream of being a website. Please star the parse-server repo on GitHub!');
res.render('splash');
});
app.get('/login', function(req, res) {
// res.status(200).send('I dream of being a website. Please star the parse-server repo on GitHub!');
res.render('login');
});
app.get('/quote/index', function(req, res) {
// res.status(200).send('I dream of being a website. Please star the parse-server repo on GitHub!');
// res.render('/scripts/index');
res.render('index', function(err, html) {
res.send(html);
});
});
// app.get('/email', function(req, res){
// res.send('testing');
// sendgrid.send({
// to: 'ankiewicz84@gmail.com',
// from: 'other@example.com',
// subject: 'Hello World next test',
// text: 'next test My first email through SendGrid'
// });
// });
//receive form information
app.post('/quote', function(req, res) {
res.send('POST request to the homepage' + req + res);
var name = req.body.name;
var html = 'Hello: ' + name + '.<br>' +
'<a href="/">Try again.</a>';
res.send(html);
// req.send('testing');
// sendgrid.send({
// to: 'ankiewicz84@gmail.com',
// from: 'other@example.com',
// subject: 'Hello World next test',
// text: 'next test My first email through SendGrid'
// });
});
// There will be a test page available on the /test path of your server url
// Remove this before launching your app
app.get('/test', function(req, res) {
res.sendFile(path.join(__dirname, '/public/test.html'));
});
var port = process.env.PORT || 1337;
var httpServer = require('http').createServer(app);
httpServer.listen(port, function() {
console.log('parse-server-example running on port ' + port + '.');
});
// This will enable the Live Query real-time server
ParseServer.createLiveQueryServer(httpServer);