Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial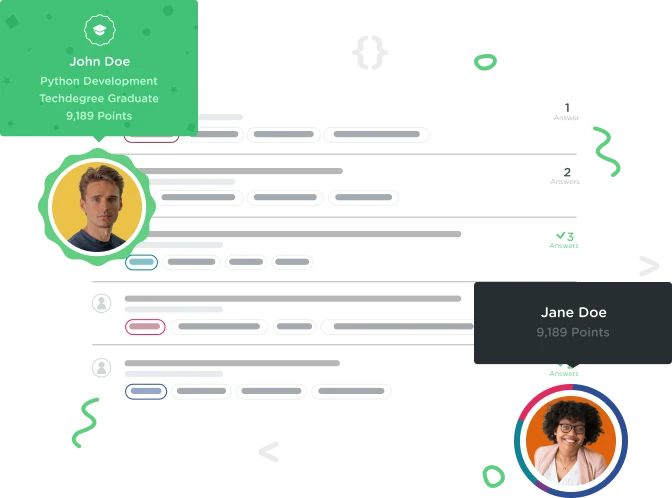

Tim Morgan
9,392 PointsRecursion
As in other programming languages, I just want to be clear that these are examples of recursive logic?
5 Answers
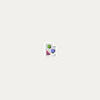
doesitmatter
12,885 PointsNope, a recursive function calls itself. In the video it is described how you can pass a function (somthing) => {console.log(something);}
inside of another function: window.setTimeOut()
.
A recursive function is something like:
let recursiveFunction = (n) => {
//stop condition
if(n === 1 || n === 2){
return 1;
}
//call
return recursiveFunction (n - 2) + recursiveFunction (n - 1);
}
for(let i = 1; i < 20; i ++){
document.write("<p>fib(" + i +"): " + recursiveFunction(i) + "</p>");
}
This writes out all the fibonacci numbers from 1 to 20.

Lucas Sacramento
912 PointsRecursion is a technique for iterating over an operation by having a function call itself repeatedly until it arrives at a result. So, for this example, recursion is not being used. It's a self executing function which receives something = "Greetings, everyone!" as parameter.
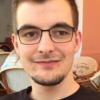
tobiaskrause
9,160 Pointsfunction recurse(depth)
{
try
{
return recurse(depth + 1);
}
catch(ex)
{
return depth;
}
}
var maxRecursion = recurse(1);
document.write("Recursion depth on this system is " + maxRecursion);
Have fun :D

Tim Morgan
9,392 PointsAhhh.. sweet clarity. Thanks so much, I guess I'm confusing the 'calling within' technique with the iteration calling itself repeatedly, although the concepts seem may seem somewhat connnected, they are fundamentally different! Thanks
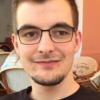
tobiaskrause
9,160 PointsAhhh man sorry i missed that "Watch Vdieo" Button on the top^^
I thought you wanted just an example of recursion. I should not answer questions when I am out of time

Tim Morgan
9,392 PointsNaw, it's still good - I am trying to get past being stuck in the concepts, and just apply the programming logic. It's 'easy' but it's complicated to sort through sometimes, anyway I tried your example, and it helps a lot!