Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial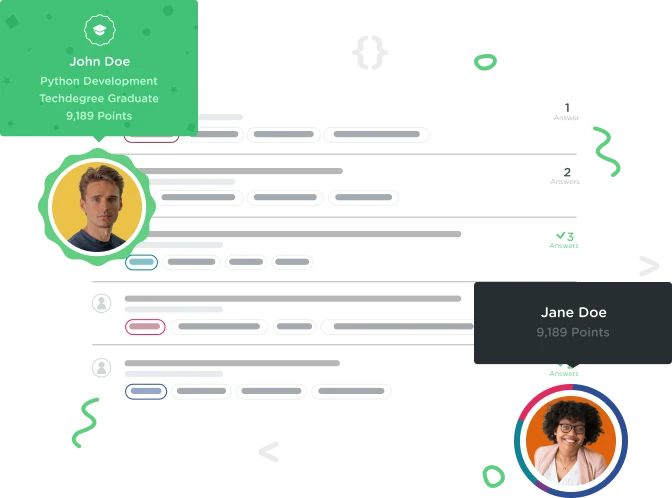

Terrell Stewart
Courses Plus Student 4,228 PointsRecursion error when attempting to log out
Within my "layout.html" file I have a url_for with that redirects to the log out view if the user is already logged in, but once I go to this view I get a jinga error of:
RecursionError: maximum recursion depth exceeded in comparison What does this mean and how can I solve this issue?
<!DOCTYPE html>
<html class="no-js">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1">
<title>{% block title %}TwoCans{% endblock %}</title>
<meta name="description" content="">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="/static/css/normalize.min.css">
<link rel="stylesheet" href="/static/css/main.css">
<!--[if lt IE 9]>
<script src="//html5shiv.googlecode.com/svn/trunk/html5.js"></script>
<script>window.html5 || document.write('<script src="/static/js/vendor/html5shiv.js"><\/script>')</script>
<![endif]-->
</head>
<body>
<header>
<div class="row">
<div class="grid-33">
<a href="{{ url_for('index') }}" class="icon-logo"></a>
</div>
<div class="grid-33">
<!-- Say Hi -->
<h1>Hello{% if current_user.is_authenticated() %} {{ current_user.username }}{% endif %}!</h1>
</div>
<div class="grid-33">
<!-- Log in/Log out -->
{% if current_user.is_authenticated() %}
<a href="{{ url_for('logout_user') }}" class="icon-power" title="Log out"></a>
{% else %}
<a href="{{ url_for('login') }}" class="icon-power" title="Log in"></a>
<a href="{{ url_for('register') }}" class="icon-profile" title="Register"></a>
{% endif %}
</div>
</div>
</header>
<!-- Flash messages -->
{% with messages = get_flashed_messages(with_categories=True) %}
{% if messages %}
{% for category, message in messages %}
<div class="notification {{ category }}">{{ message }}</div>
{% endfor %}
{% endif %}
{% endwith %}
<div class="row">
<div class="main">
<nav>
<a href="{{ url_for('index') }}">All</a>
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<div class="row">
<p>A Social App built with Flask<br>by <a href="http://teamtreehouse.com">Treehouse</a></p>
</div>
</footer>
<script src="/static/js/vendor/disTime.min.js"></script>
<script src="/static/js/main.js"></script>
</body>
</html>
from flask import (Flask, g,render_template,flash,redirect,url_for)
from flask.ext.bcrypt import check_password_hash
from flask.ext.login import LoginManager,login_user,logout_user,login_required#Have to be logged in to use this view
import models #File created
import forms #File created
DEBUG = True
PORT = 8000
HOST = '0.0.0.0'
app = Flask(__name__)
app.secret_key = 'rfvreg5tgb45tg5re'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login' #Login refers to the def login() function
@login_manager.user_loader
def load_user(userid):
try:
return models.User.get(models.User.id==userid) #Find out what user id is
except models.DoesNotExist:
return None
@app.before_request
def before_request():
"""Connect to database before each request."""
g.db = models.DATABASE
g.db.connect()
@app.after_request
def after_request(response):
"""Close the database connection after each request"""
g.db.close()
return response
@app.route('/register',methods=('GET','POST'))
def register():
form = forms.RegisterForm()
if form.validate_on_submit():
flash("Yay you registered!", "success")
models.User.create_user(
username = form.username.data,
email = form.email.data,
password = form.password.data
)
return redirect(url_for('index'))
return render_template('register.html',form=form)
@app.route('/login',methods=('GET','POST'))
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.get(models.User.email == form.email.data)
except models.DoesNotExist:
flash("Your email or password doesn't match!","error")
else:
if check_password_hash(user.password, form.password.data):
login_user(user)
flash("You've been logged in!","success")
return redirect(url_for('index'))
else:
flash("your email or password doesn't match!", "error")
return render_template('login.html', form=form) #Why do we use form=form???? FIND OUT
@app.route('/logout')
@login_required #You must be logged if not you will get rediredted to the log in page
def logout_user():
logout_user() #Deletes the login_user(user) cookie in the log in view
flash("You've been logged out! Come back soon!","success")
return redirect(url_for('index'))
@app.route('/')
def index():
return'Hey'
if __name__ == '__main__':
models.initialize()
try:
models.User.create_user(
username='Terrell Stewart',
email='terrell.p.stewart@gmail.com',
password='password',
admin=True
)
except ValueError :
pass
app.run(debug=DEBUG,host=HOST,port=PORT)
[MOD: added ```python formatting -cf]
1 Answer
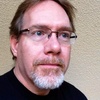
Chris Freeman
Treehouse Moderator 68,423 PointsThe first line of the logout()
calls the logout() this will recursive call itself until the recursive limit is reached.
def logout_user():
logout_user() #Deletes the login_user(user) cookie in the log in view
Perhaps you meant to call the view "logout"