Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial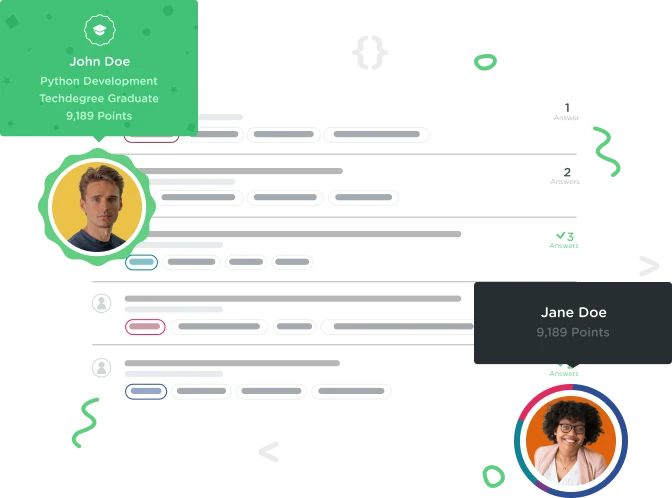

Ireneusz Kopta
6,212 Pointsrecursion in javascript
cant really wrap my head around it. So:
var power = function(base, exponent) {
if (exponent === 0) {
return 1;
} else {
return base * power(base, exponent - 1);
}
};
I guess it works this way:
base = 2
exponent = 5
exponent !== 0 so base case is skipped
return 2 * power(2, 4)
exponent = 4
return 2 * power(2, 3)
exponent = 3
return 2 * power(2, 2)
exponent = 2
return 2 * power(2, 1)
exponent = 1
return 2 * power(2, 0)
exponent = 0
return 1
but when return 2* power(2,4) is running should not power * 2 and then * 4 as it is in between brackets?
4 Answers
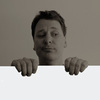
Sean T. Unwin
28,690 PointsTo expand upon what Ryan Gordon said and to help you understand better what is going on with this function, you can copy the below code, open your browser Dev Tools' console, execute the code and see what is happening.
var result = 0;
var base = 2;
var exponent = 5;
var power = function(base, exponent) {
var r = 0;
// output the value of exponent
console.log("exponent: " + exponent);
if ((exponent === 0) || (typeof exponent !== "number")) {
console.info(exponent); // this should output 0;
r = 1;
} else {
r = base * power(base, exponent - 1);
}
// output the value of r
console.log("r: " + r);
return r;
};
result = power(base, exponent);
// output the final value (result)
console.log("result: " + result);
The above should output the following:
"exponent: 5"
"exponent: 4"
"exponent: 3"
"exponent: 2"
"exponent: 1"
"exponent: 0"
: 0
"r: 1"
"r: 2"
"r: 4"
"r: 8"
"r: 16"
"r: 32"
"result: 32"
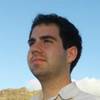
Ryan Gordon
1,340 PointsIt looks like you're not finishing the recursion. You've gone through all the if statements but the function calls are not complete until after 1 is returned.
After the return 1 happens the function call power(2,1) can be finished returning 2, then power(2,2) returning 4, then power(2,3) returns 8, then power(2,4) returns 16, then power(2,5) can return the final answer 32.

Ireneusz Kopta
6,212 Pointsbut how the 2 * power(2, 4) works for example. I thought it should : 2* 2 // 2 * base 2* 4 // 2* exponent .
how come its not multiplying exponent?
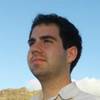
Ryan Gordon
1,340 PointsLet me write it this way, hope this helps:
power(2,5) = 2 * power(2,4) = 2 * 2 * power(2,3) = 2 * 2 * 2 * power(2,2) = 2 * 2 * 2 * 2 * power(2,1) = 2 * 2 * 2 * 2 * 2 * power(2,0) = 2 * 2 * 2 * 2 * 2 * 1
You call power(2,5), but each recursive call is not finished until the final call that returns 1. That is to say the function is being executed 5 times, then 1 is returned as a result of power(2,0). Then the function finishes the call to power(2,1) then it can finish the call to power(2,2) etc.
Once it get's to power(2,5) it knows the result to power(2,4), see above, so it can return the answer of 2*power(2,4).

Ireneusz Kopta
6,212 PointsI understand that bit. but i dont understand why when we calling return base * power(base, exponent - 1);
it only multiply base * base but not multiplying base time exponent witch is next to base after the come between the brackets.
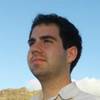
Ryan Gordon
1,340 PointsWhen you're calculating a power you are just multplying a base by itself exponent many times. So the only number that is ever multiplied, in this example, is 2. The exponent is the number of times you want to multiply the base by itself.
base = 3, exponent =2
3*3
base = 9, exponent = 3
9*9*9
base = 2, exponent = 5
2*2*2*2*2
Hope that helps.