Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial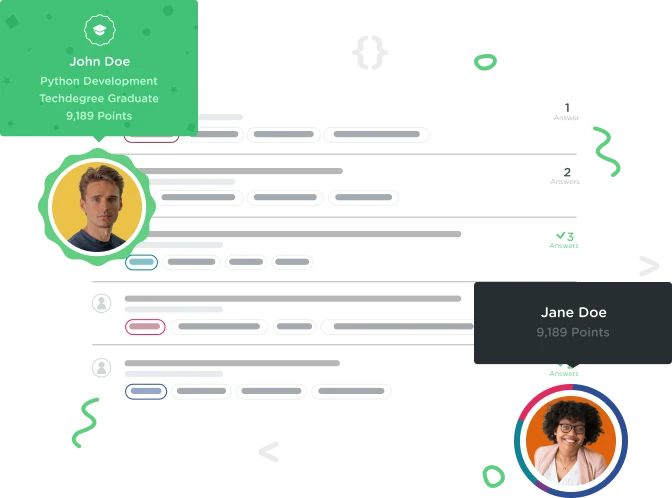

Fareez Ahmed
12,728 PointsRecursion Practice
I'm trying to practice recursion by building a function that puts each element of a list into it's own list, but I'm getting stuck. where am I going wrong?
function ownList(arr){
if (arr.length >= 1) {
arr[0] = [arr[0]];
return;
} else {
return arr[0].concat(ownList(arr.slice(1)));
}
}
var arr = [1,2,3]
console.log(ownList(arr))// returns []
//should return [[1],[2],[3]]
1 Answer
Seth Shober
30,240 PointsI'm a little confused as to what you are trying to do/achieve. Could you be a little more explicit? Here is a classic example I put together for recursion and fizzbuzz which may turn on the lightbulb for you. Please note that though this is a valid way to do this, I may not choose to use recursive in this situation vs more traditional conditionals like if...else
, ternary
, or switch
.
function fizzBuzzRecursive(start, end) {
// base check
if (start > end || typeof start != 'number' || typeof end != 'number') return
// do fizzbuzz
if ( start % 3 == 0 && start % 5 == 0 ) console.log('FizzBuzz')
else if ( start % 3 == 0 ) console.log('Fizz')
else if ( start % 5 == 0 ) console.log('Buzz')
else console.log(start)
// call recursively
fizzBuzzRecursive(start + 1, end) // cannot use ++
}
Here is a jsperf showing comparisons if interested.