Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial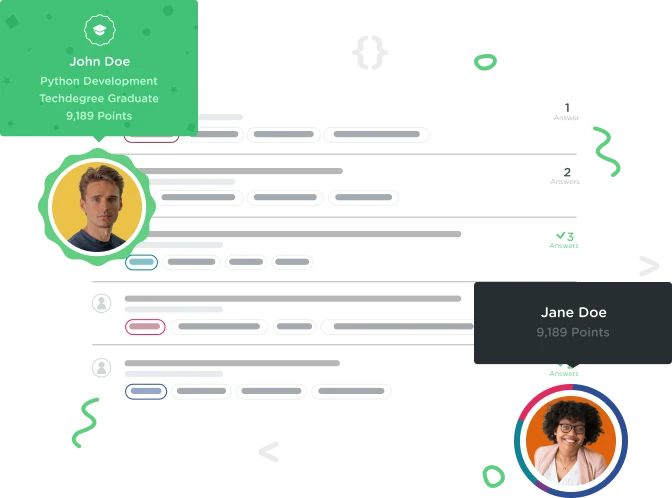
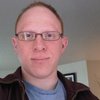
Joseph Damiani
1,066 PointsRedirect from thank you page.
I have successfully created a thank you page after a form submission without any trouble. My question is how do you redirect from the thank you page back to the contact page after 5 seconds?
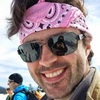
Jack McDowell
37,797 PointsThe problem could be that header() requires an absolute uri, or that code is being output before it. I'm not sure if a url like
header('Refresh: 10; url=index.php');
would fix it, but you can read more about the options here.
And here for the manual that references header being executed before any other html code.
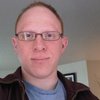
Joseph Damiani
1,066 PointsApparently this worked and the code was passed by the validator. While this feels like a rogue method it worked. Thanks for your help in the placement of the code for the refresh.
<?php if (isset($_GET["status"]) AND $_GET["status"] == "thanks") { ?>
<meta http-equiv="refresh" content="5;URL='contact.php'" />
<p>Thanks for the email! I’ll be in touch shortly!</p>
1 Answer
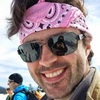
Jack McDowell
37,797 PointsHello, for my contact page (contact/index.php) I can do that by adding:
header('Refresh: 5; index.php');
after it sends the email. I'll post the code for my contact index.php so that you can see where it goes (it's on line 87).
<?php
require_once("../inc/config.php");
/* This file contains instructions for three different states of the form:
* - Displaying the initial contact form
* - Handling the form submission and sending the email
* - Displaying a thank you message
*/
// a request method of post indicates that
// we are receiving a form submission
if ($_SERVER["REQUEST_METHOD"] == "POST") {
// the form has fields for name, email, and message
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
$message = trim($_POST["message"]);
// the fields name, email, and message are required
if ($name == "" OR $email == "" OR $message == "") {
$error_message = "You must specify a value for name, email address, and message.";
}
// this code checks for malicious code attempting
// to inject values into the email header
if (!isset($error_message)) {
foreach( $_POST as $value ){
if( stripos($value,'Content-Type:') !== FALSE ){
$error_message = "There was a problem with the information you entered.";
}
}
}
// the field named address is used as a spam honeypot
// it is hidden from users, and it must be left blank
if (!isset($error_message) && $_POST["address"] != "") {
$error_message = "Your form submission has an error.";
}
require_once(ROOT_PATH . "inc/phpmailer/class.phpmailer.php");
$mail = new PHPMailer();
if (!isset($error_message) && !$mail->ValidateAddress($email)){
$error_message = "You must specify a valid email address.";
}
// if, after all the checks above, there is no message, then we
// have a valid form submission; let's send the email
if (!isset($error_message)) {
$email_body = "";
$email_body = $email_body . "Name: " . $name . "<br>";
$email_body = $email_body . "Email: " . $email . "<br>";
$email_body = $email_body . "Message: " . $message;
$mail->SetFrom($email, $name);
$address = "orders@shirts4mike.com";
$mail->AddAddress($address, "Shirts 4 Mike");
$mail->Subject = "Shirts 4 Mike Contact Form Submission | " . $name;
$mail->MsgHTML($email_body);
// if the email is sent successfully, redirect to a thank you page;
// otherwise, set a new error message
if($mail->Send()) {
header("Location: " . BASE_URL . "contact/?status=thanks");
exit;
} else {
$error_message = "There was a problem sending the email: " . $mail->ErrorInfo;
}
}
}
?><?php
$pageTitle = "Contact Mike";
$section = "contact";
include(ROOT_PATH . 'inc/header.php'); ?>
<div class="section page">
<div class="wrapper">
<h1>Contact</h1>
<?php // if status=thanks in the query string, display an thank you message instead of the form ?>
<?php if (isset($_GET["status"]) AND $_GET["status"] == "thanks") {
header('Refresh: 5; index.php');?>
<p>Thanks for the email! I’ll be in touch shortl !</p>
<?php } else { ?>
<?php
if (!isset($error_message)) {
echo '<p>I’d love to hear from you! Complete the form to send me an email.</p>';
} else {
echo '<p class="message">' . $error_message . '</p>';
}
?>
<form method="post" action="<?php echo BASE_URL; ?>contact/">
<table>
<tr>
<th>
<label for="name">Name</label>
</th>
<td>
<input type="text" name="name" id="name" value="<?php if (isset($name)) { echo htmlspecialchars($name); } ?>">
</td>
</tr>
<tr>
<th>
<label for="email">Email</label>
</th>
<td>
<input type="text" name="email" id="email" value="<?php if(isset($email)) { echo htmlspecialchars($email); } ?>">
</td>
</tr>
<tr>
<th>
<label for="message">Message</label>
</th>
<td>
<textarea name="message" id="message"><?php if (isset($message)) { echo htmlspecialchars($message); } ?></textarea>
</td>
</tr>
<tr style="display: none;">
<?php // the field named address is used as a spam honeypot ?>
<?php // it is hidden from users, and it must be left blank ?>
<th>
<label for="address">Address</label>
</th>
<td>
<input type="text" name="address" id="address">
<p>Humans (and frogs): please leave this field blank.</p>
</td>
</tr>
</table>
<input type="submit" value="Send">
</form>
<?php } ?>
</div>
</div>
<?php include(ROOT_PATH . 'inc/footer.php') ?>
Joseph Damiani
1,066 PointsJoseph Damiani
1,066 PointsThis will only work on my localhost but it won't work when uploaded to my website. Any suggestions on what I am over looking?