Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial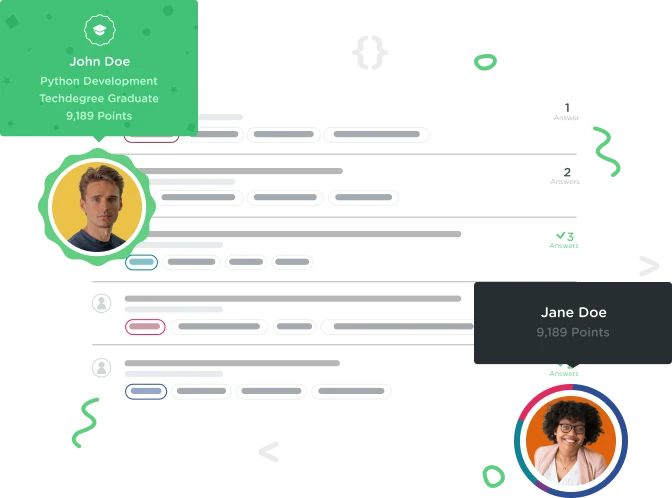
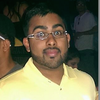
Mathew Kurian
4,698 PointsReducing Complexity of Guessing Game
I'm currently learning about complexity, efficiency, and O(n) of programs. It's pretty hard to start learning, and I'm trying to wrap my head around it. Most well-know companies like Google or Facebook, require you to have a robust understanding of algorithm analysis.
Can you guys take a look at my program for the guessing game, and tell me how complex it is? Is there anywhere where I am needlessly making it very complex? How can I make it more efficient?
import random
key = random.randint(1,10)
maxattempt = 3
attempts = 0
print("***************************************************")
print("******** WELCOME TO MATHEW'S GUESSING GAME!********")
print("***************************************************")
print("A random number between 1 and 10 has been chosen. (Including 1 and 10!)")
print("You must guess this number. You have three chances to do so.")
input("Are you ready?")
print("Then let us begin.")
choice = 1
while 0<choice<11:
print("You have guessed {} times. You have {} attempts left.".format(attempts, maxattempt-attempts))
user = input("Guess the number: ")
choice = int(user)
if choice < key:
print("Your guess is too low. Guess higher.")
attempts += 1
if attempts == maxattempt:
print("You have run out of tries!")
print("The chosen number was {}.".format(key))
print("GAME OVER!")
print("My condolences. Better luck next year.")
break
continue
elif choice > key:
print("Your guess is too high. Guess again.")
attempts += 1
if attempts == maxattempt:
print("You have run out of tries!")
print("The chosen number was {}.".format(key))
print("GAME OVER!")
print("My condolences. Better luck next year.")
break
continue
elif choice == key:
print("Congratulations you guessed the number!")
print("The chosen number was {}!".format(key))
break
print("Hope you enjoyed the game!")
Also if anyone has any good resources of guides to learn algorithm analysis for programming, please let me know!
1 Answer

Matthew Carr
11,220 PointsOne thing, you've duplicated the code to check if they have reached their maximum amount of guesses. Why not make a function that does that and call it each time?
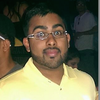
Mathew Kurian
4,698 PointsHow would I go about doing that? Do you mean just create another function with all the print statements together from that if clause put together? Or do you mean create a function that actually checks equality on max attempts?
Mathew Kurian
4,698 PointsMathew Kurian
4,698 PointsI have no idea why some of my code is grey, it works fine in the Python workspace.