Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial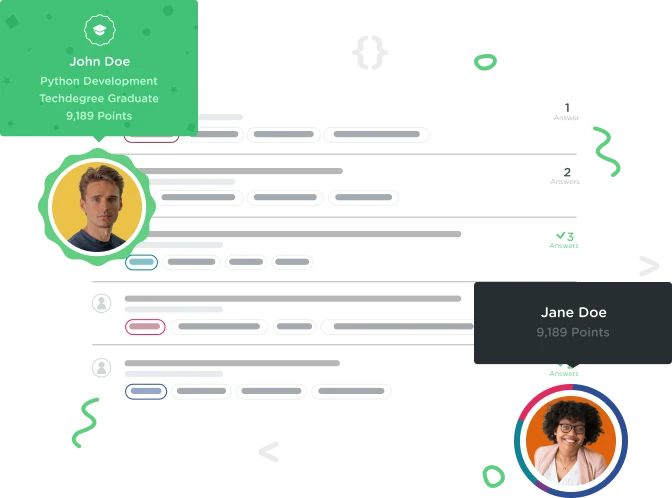

Nelson Lourenco
1,766 PointsReducing var color to single line
How come the following code won't work in my script?
function rgbColor() {
var color = 'rgb(' + randomRGB() + ',' + randomRGB() + ',' + randomRGB() + ')';
return color;
}
I would of thought you can simply produce a single CSS expression representing var color.
3 Answers

Saskia Lund
5,673 PointsWell on first glance, I'd say, try adding the rgbColor function parenthesis and the semi colon of that inline CSS to your for loop:
for (var i = 0; i < 10; i += 1) {
colorBall += '<div style="background-color:' + rgbColor() + ';"></div>';
}

Saskia Lund
5,673 PointsWhat is the content of the randomRGB() function? You can return the rgb function like so.. without a function scope variable:
function randomRGB() {
return Math.round(Math.random() * 255);
}
function rgbColor() {
return 'rgb(' + randomRGB() + ', ' + randomRGB() + ', ' + randomRGB() + ')';
}

Nelson Lourenco
1,766 PointsRight, I did specify a randomRGB function but it still doesn't output. I should of pasted my entire program, here it is:
var colorBall = '';
var rgbColor;
function randomRGB() {
Math.floor(Math.random() * 256 );
}
function rgbColor() {
var color = 'rgb(' + randomRGB() + ',' + randomRGB() + ',' + randomRGB() + ')';
return color;
}
for (var i = 0; i < 10; i += 1) {
colorBall += '<div style="background-color:' + rgbColor + '"></div>';
}
document.write(colorBall);
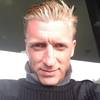
Rasmus Rønholt
Courses Plus Student 17,358 PointsHi Nelson
If you never did find out what was wrong here then here goes. You declare a variable rgbColor at the top of your code. You don't use it for anything thouh, and when it comes time to create the html, you insert that (empty) variable instead of calling the function rgbColor() - note the paranthesis :)

Clara Roldan
3,074 PointsRasmus is right but also note that your randomRGB() function isn't returning anything... You are creating a random color but never asking the function to return it.
function randomRGB() {
return Math.floor(Math.random() * 256 );
}