Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial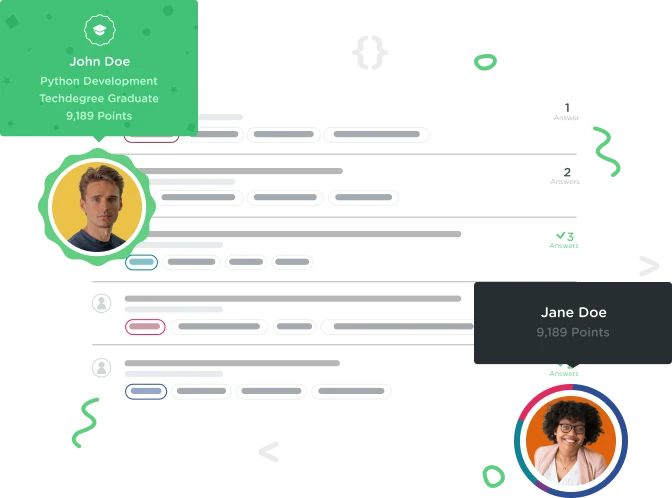

David Gabriel
Python Web Development Techdegree Student 979 Pointsrefactor
Hi all, is there anyone assist with the error on the following challenges:
def main ()
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
make a list to hold onto our items
shopping_list = []
for item in shopping_list:
print(item)
while True: # ask for new items new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
def add_to_list(shopping_list, new_item): # add new items to our list shopping_list.append(new_item) print("Added {}. List now has {} items.".format(new_item, len(shopping_list))) return shopping_list show_list(shopping_list) def show_list(shopping_list): # print out the list print("Here's your list:")
def main ()
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
# make a list to hold onto our items
shopping_list = []
for item in shopping_list:
print(item)
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
show_list(shopping_list)
def show_list(shopping_list):
# print out the list
print("Here's your list:")
5 Answers
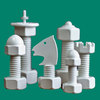
Steven Parker
231,269 PointsFor this challenge, all you do is move code that is not already part of a function into a new function. So the only new code you need to write is the "def" line for the function, everything else just needs to be properly indented to become part of the function.
Any code already in a function does not need to be changed or moved at all.
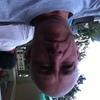
Stuart McIntosh
Python Web Development Techdegree Graduate 22,874 PointsHi there, your code was fine just need a slight refactoring - hopefully the below makes sense
# make a list to hold onto our items
# this variable has a global scope so no need to pass it around
shopping_list = []
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def add_to_list(new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return
def show_list():
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def main():
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list()
continue
add_to_list(new_item)
# the if state below is a special command in a python
# when python sees this it executes anything below first.
if __name__ == '__main__':
main()

David Gabriel
Python Web Development Techdegree Student 979 PointsHi Stuart, I have refactor the as you said but did not pass; amazingly I run it on jupyter note book and was well excuted. I don't know what to do?sorry for bagging again.
make a list to hold onto our items
this variable has a global scope so no need to pass it around
shopping_list = []
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def add_to_list(new_item): # add new items to our list shopping_list.append(new_item) print("Added {}. List now has {} items.".format(new_item, len(shopping_list))) return
def show_list(): # print out the list print("Here's your list:") for item in shopping_list: print(item)
def main(): while True: # ask for new items new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list()
continue
add_to_list(new_item)
the if state below is a special command in a python
when python sees this it executes anything below first.
if name == 'main': main()
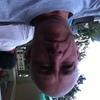
Stuart McIntosh
Python Web Development Techdegree Graduate 22,874 PointsHi there, no problem. Couple of questions - what are running this in? via a command prompt, or an IDE like sublime or in Treehouse workspaces? Also, what is your version of Python? you can check this via a command prompt (on mac) type in : python --version. If this comes back with 2.7 I suggest you update to the latest 3.6.
p.s. if name == 'main': main() this is incorrect.
if __name__ == '__main__':
main()
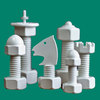
Steven Parker
231,269 PointsThis is for a course challenge (see the button in the upper right ). The challenge provides a simulated Python environment.

David Gabriel
Python Web Development Techdegree Student 979 Pointshi Stuart, I am using 3.6 version and it was well executed but did not pass on treehouse shell. i will try again almost three days with not success
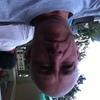
Stuart McIntosh
Python Web Development Techdegree Graduate 22,874 PointsI am super sorry - I didnt read the challenge - this works:
def show_help():
# print out instructions on how to use the app
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def show_list(shopping_list):
# print out the list
print("Here's your list:")
for item in shopping_list:
print(item)
def add_to_list(shopping_list, new_item):
# add new items to our list
shopping_list.append(new_item)
print("Added {}. List now has {} items.".format(new_item, len(shopping_list)))
return shopping_list
def main():
show_help()
# make a list to hold onto our items
shopping_list = []
while True:
# ask for new items
new_item = input("> ")
# be able to quit the app
if new_item == 'DONE':
break
elif new_item == 'HELP':
show_help()
continue
elif new_item == 'SHOW':
show_list(shopping_list)
continue
add_to_list(shopping_list, new_item)
show_list(shopping_list)
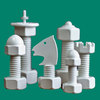
Steven Parker
231,269 Points FYI: According to a moderator, explicit answers without any explanation are strongly discouraged by Treehouse.
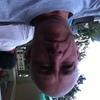
Stuart McIntosh
Python Web Development Techdegree Graduate 22,874 Pointsok... apologies again.
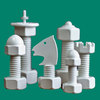
Steven Parker
231,269 PointsNo need to apologize .. just add an explanation or delete it.
David Gabriel
Python Web Development Techdegree Student 979 PointsDavid Gabriel
Python Web Development Techdegree Student 979 PointsHi steve, still not passing, but manage to execute it on Jupyter note book; not quite sure whats going on?
make a list to hold onto our items
this variable has a global scope so no need to pass it around
shopping_list = []
def show_help(): # print out instructions on how to use the app print("What should we pick up at the store?") print("""
Enter 'DONE' to stop adding items. Enter 'HELP' for this help. Enter 'SHOW' to see your current list. """)
def add_to_list(new_item): # add new items to our list shopping_list.append(new_item) print("Added {}. List now has {} items.".format(new_item, len(shopping_list))) return
def show_list(): # print out the list print("Here's your list:") for item in shopping_list: print(item)
def main(): while True: # ask for new items new_item = input("> ")
the if state below is a special command in a python
when python sees this it executes anything below first.
if name == 'main': main()
Steven Parker
231,269 PointsSteven Parker
231,269 PointsFor the spacing to show up right, you need to use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
But I still think you're doing too much. To pass this challenge, the only new code you need to add is the "def" line for the new "main" function. Then you just change indentation on the following lines.