Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial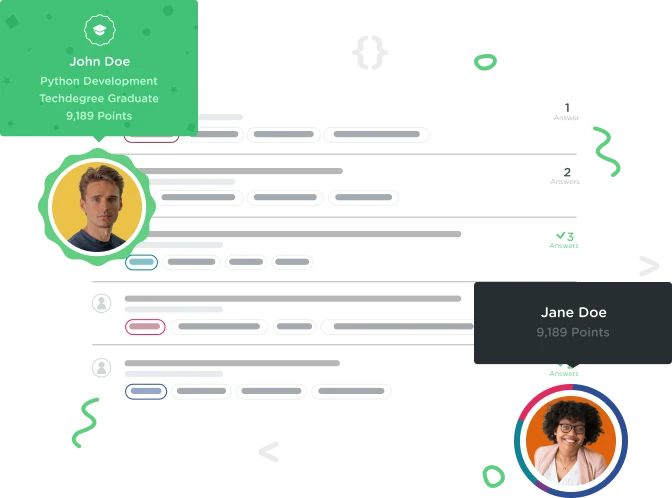

Conary Beckford
Full Stack JavaScript Techdegree Student 5,046 PointsRefactor 2
document.addEventListener('DOMContentLoaded', () => {
const form = document.getElementById('registrar');
const input = form.querySelector('input');
const mainDiv = document.querySelector('.main');
//const ul...
const ul = document.getElementById('invitedList');
const div = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckBox = document.createElement('input');
filterLabel.textContent = "Hide those who haven't responded";
filterCheckBox.type = 'checkbox';
div.appendChild(filterLabel);
div.appendChild(filterCheckBox);
mainDiv.insertBefore(div, ul);
filterCheckBox.addEventListener('change', (e) =>{
const isChecked = e.target.checked;
const lis = ul.children;
if (isChecked) {
for (let i = 0; i < lis.length; i += 1) {
let li = lis[i];
if (li.className === 'responded') {
li.style.display = '';
}else {
li.style.display = 'none';
}
}
} else {
for (let i = 0; i < lis.length; i += 1) {
let li = lis[i];
li.style.display = '';
}
}
});
function createLI(text) {
function createElement(elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
function appendToLI(elementName, property, value) {
const element = createElement(elementName, property, value);
li.appendChild(element);
return element;
}
//const ul = document.getElementById('invitedList');
const li = document.createElement('li');
appendToLI('span', 'textContent', text);
//span.textContent = text;
//deleted because appendToLI is now taking care of --li.appendChild(span);
const label = appendToLI('label', 'textContent', 'Confirmed');
//label.textContent = 'Confirmed';
const checkbox = createElement('input', 'type', 'checkbox');
// checkbox.type = 'checkbox';
label.appendChild(checkbox);
//li.appendChild(label);
appendToLI('button', 'textContent', 'edit');
//editButton.textContent = 'edit';
//appendToLI noW takes care of ---li.appendChild(editButton);
appendToLI('button', 'textContent', 'remove');
//removeButton.textContent = 'remove';
//appendToLI Now takes care of ---li.appendChild(removeButton);
return li;
}
form.addEventListener('submit', (e) => {
e.preventDefault();
// console.log(input.value);
const text = input.value;
input.value = '';
//const ul moved to top of file so it can be globally accessible.
const li = createLI(text);
ul.appendChild(li);
});
//true(checked) and false(unchecked)
ul.addEventListener('change', (e) => {
//console.log(e.target.checked);
const checkbox = event.target;
const checked = checkbox.checked;
const listItem = checkbox.parentNode.parentNode;
if (checked) {
listItem.className = 'responded';
} else {
listItem.className = '';
}
});
ul.addEventListener('click', (e) => {
if (e.target.tagName === 'BUTTON') {
const button = e.target;
const li = button.parentNode;
const ul = li.parentNode;
const action = button.textContent;
const nameActions = {
remove: () => {
ul.removeChild(li);
},
edit: () => {
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'save';
},
save: () => {
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'edit';
}
};
// select and run action in button's name
nameActions[action]();
}
});
Moderator edited: Markdown corrected so that code renders properly in the forums. -jn
Can someone tell me why this is not running in my editor?

Conary Beckford
Full Stack JavaScript Techdegree Student 5,046 PointsHere is the link to my snapshot.
2 Answers
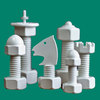
Steven Parker
231,269 PointsApparently, the outer "addEventListener" function is not closed. The unusual indentation makes it hard to spot (but testing in the workspace revealed the issue quickly).
The code needs one more ending brace and parenthesis:
});

Conary Beckford
Full Stack JavaScript Techdegree Student 5,046 PointsThank you. I'm semi new to the functionality of the workspace. How can I run a test in it to show errors?
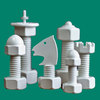
Steven Parker
231,269 PointsWhile running the preview, I just opened my browser's development tools.
Since you're already taking the prerequisite course, once you finish you might enjoy the Debugging JavaScript in the Browser workshop.

Conary Beckford
Full Stack JavaScript Techdegree Student 5,046 PointsI will study the workshop you've suggested. Thank you.
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThis code depends on an HTML component that is not shown here.