Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial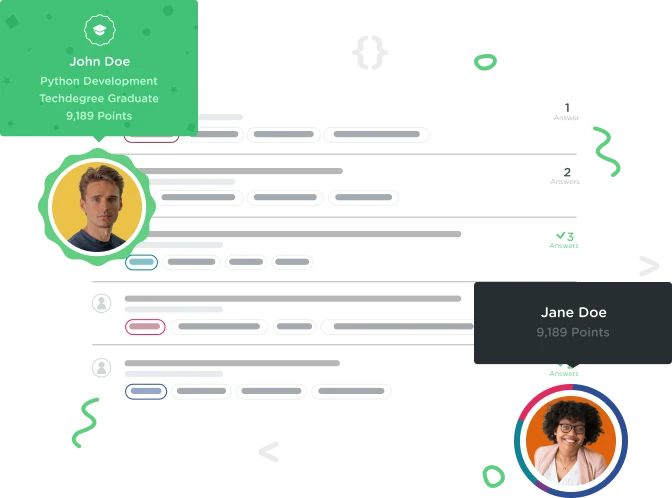

Shadab Khan
5,470 PointsRefactoring the Console Calculator
Hi Guys,
I have written a console calculator program that takes two numbers and an operator as an input from the end user and displays the operation result. The program works well; however, I interested to see how can we refactor it any further ? Below is my version of the code. Look forward to hear from you guys.
using System;
namespace ConsoleCalculator
{
class Program
{
static void Main(string[] args)
{
//Prompt user to enter first number, operator, and second number until "quit" is entered.
while (true)
{
double firstNumber = 0.0;
double secondNumber = 0.0;
double result = 0.0;
string firstEntry, secondEntry;
char operation = '\0';
Console.Write("Enter the first number : ");
firstEntry = Console.ReadLine();
if (firstEntry.ToLower() == "quit")
{
break;
}
Console.Write("Enter the second number : ");
secondEntry = Console.ReadLine();
if(secondEntry.ToLower() == "quit")
{
break;
}
try
{
firstNumber = double.Parse(firstEntry);
secondNumber = double.Parse(secondEntry);
}
catch (Exception)
{
Console.WriteLine("Please enter a valid number for operation.");
continue;
}
Console.Write("Enter the operator : ");
operation = char.Parse(Console.ReadLine());
//Begin operations.
if (operation == '+')
{
result = firstNumber + secondNumber;
}
else if(operation == '-')
{
result = firstNumber - secondNumber;
}
else if (operation == '/')
{
result = firstNumber / secondNumber;
}
else
{
result = firstNumber * secondNumber;
}
Console.WriteLine("The result of operation " + operation + " is : " + result);
}
Console.WriteLine("Goodbye!");
}
}
}
1 Answer
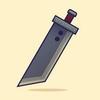
Allan Clark
10,810 PointsThe main thing I can see that would help with readability is if you refactored out the calculator logic into its own class. I would send in the raw user input into the constructor and let the Calculator class worry about the data validation. That will keep a lot of the try/catch-ing out of the main method. Generally you want to have the main method be as minimal as possible.
Also as a note, once you get the hang of Parse methods and Try/catch-ing those, you can move to the TryParse methods. They essentially encapsulate that whole process and rather than dealing with exceptions you can do a null check or just use the bool returned. The try catch block would turn into this.
double firstNumber;
if (!double.TryParse("", out firstNumber))
{
//display error
};
p.s. if you have never seen the 'out' keyword it basically means if the parse is successful it will store it in the variable you pass it.