Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial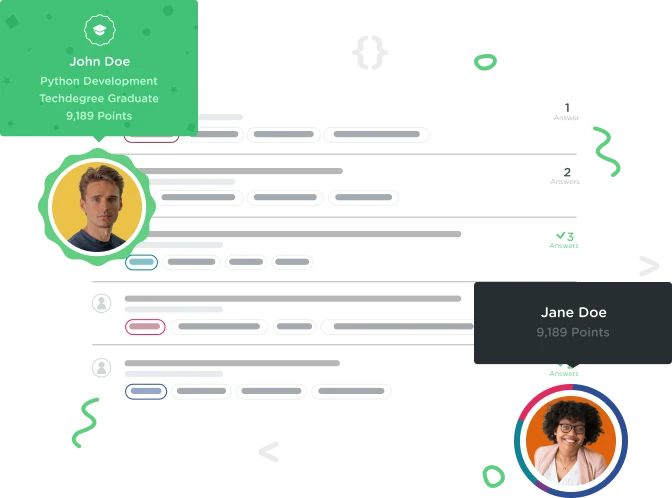
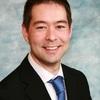
Mark Chesney
11,747 Pointsrefactoring word_count (an intermediate Python solution)
Hi everyone,
I'm revisiting this challenge as I'm finishing up the intermediate Python track, and looking for a more Pythonic solution to this, just to demonstrate what I've learned. I came up with a dictionary comprehension. Would anyone have any good feedback on whether I could slim this down sensibly? I'm really curious if the for-loop can be discarded. Much thanks in advance!
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
list_of_keys = string.lower().split()
dict = {key: 0 for key in list_of_keys}
for word in list_of_keys:
dict[word] += 1
return dict
1 Answer

kyle kitlinski
5,619 Pointssentence = "I do not like it Sam I Am"
def word_count(string):
list_of_keys = string.lower().split()
dict = {key: 0 for key in list_of_keys}
for word in list_of_keys:
dict[word] += 1
return dict
%timeit word_count(sentence)
>> 1.41 µs ± 5.79 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each)
def comprehension_count(string):
list_of_keys = string.split()
return {word: list_of_keys.count(word) for word in set(list_of_keys)}
%timeit comprehension_count(sentence)
>> 1.87 µs ± 2.11 ns per loop (mean ± std. dev. of 7 runs, 1000000 loops each)
# however if we use a larger string the comprehension count is a tad faster
%timeit word_count(sentence*999999)
>> 1.36 s ± 5.17 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
%timeit comprehension_count(sentence*999999)
>> 1.33 s ± 4.68 ms per loop (mean ± std. dev. of 7 runs, 1 loop each)
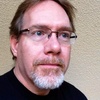
Chris Freeman
Treehouse Moderator 68,441 PointsThe comprehension solution posted is exploiting that the set(list_of_keys)
is very small since using sentence*999999
. If word_count
added the set
optimization, performance would equivalent to the comprehension:
def word_count(string):
list_of_keys = string.lower().split()
words = {key: 0 for key in set(list_of_keys)}
for word in list_of_keys:
words[word] += 1
return words
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsIt great to revisit old challenges with new skills! Be careful not to add complexity for the sake of elegance. Can you say more about what was refactored?
This new solution may be less efficient that using an
if
statement to check if word is already in thedict
. The dict comprehension is doing more work than theif
in that it is reinitializing keys each time a repeated key is reached. It's the same as: