Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial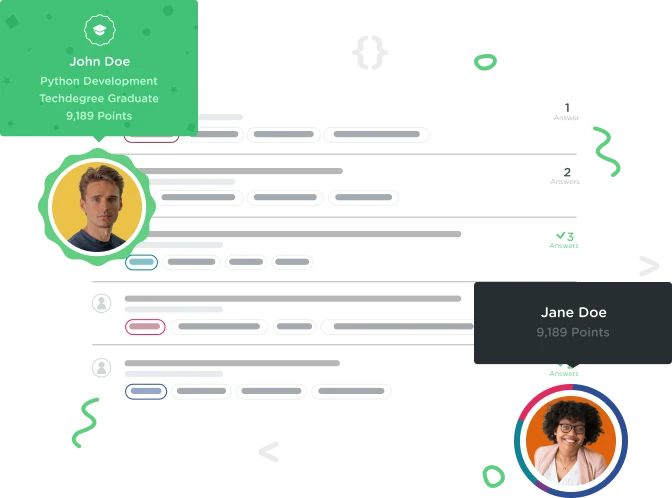
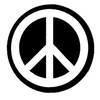
john larson
16,594 PointsReferenceError: Invalid left-hand side in assignment
var html = "";
var i;
for (i = 0; i < 10; 1 += 1) {
html += "<div>" + i + "</div>";
}
document.getElementById("print").innerHTML = html;
1 Answer
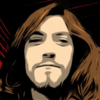
Alec Plummer
15,181 PointsYour for loop is constructed a little wompy, this should fix your problem. Define i inside of the for loop, you don't want to clutter your global scope with throw away variables. The for loop is broken down into its 3 sections; define counter, check counter against length(in this case, 10), add 1 to i(i++). Then it repeats by checking i against length(10) and adding 1 to i until i is no longer less than length.
var html = "";
for (var i = 0; i < 10; i++) {
html+= "div" + i "</div>";
}
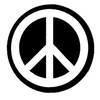
john larson
16,594 PointsAlec, thank you for your response. I'm getting mixed messages on where to declare... var i, in for loop. I know at this point in the course Dave declares it inside (). but js lint and I believe w3schools says to declare it before the loop.
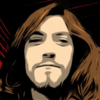
Alec Plummer
15,181 PointsNo problem. It can be confusing when you first start learning, wait until you get to objects and prototypal inheritance for the first time lol. Always declare within the loop, I've never seen var = i; declared outside of the loop in production. The reason being that you don't want to clutter the global scope if you don't have to. You wouldn't want some random variable screwing you up 100+ lines down the js file lol.
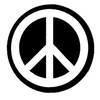
john larson
16,594 PointsOk, so them I'm thinking that the places I've seen var i; declared outside a loop, it was possibly inside a function. That way it will not muddle up the global scope?
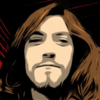
Alec Plummer
15,181 Pointscould be, as a rule of thumb though you always want to declare the counter within the loop. When looking at w3schools, keep in mind that the sample code is just to illustrate something and not necessarily how you would write that code in 'the real world' so to speak.
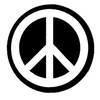
john larson
16,594 PointsWow, that makes a huge difference, cause I got the impression that their stuff was THE way to do it. So, thanks for sharing that with me. There's still the js Lint thing saying to declare var i: outside the loop. But I get the impression that Lint is not all it's cracked up to be either. Thanks again.
john larson
16,594 Pointsjohn larson
16,594 PointsI just can't see what I'm missing