Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial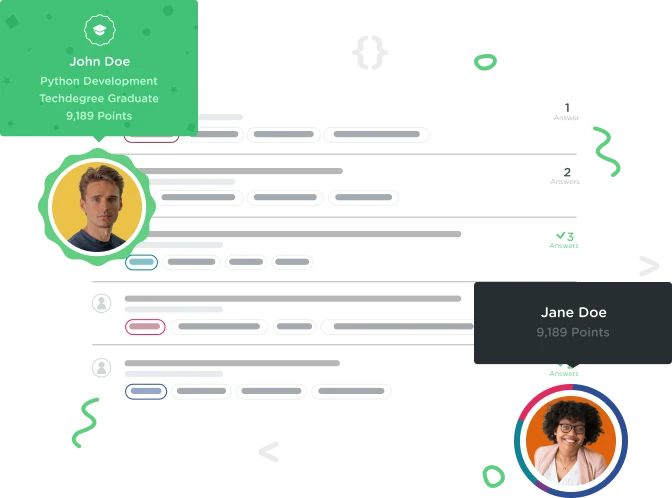
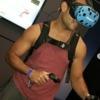
Aaron Noble
6,559 PointsReferencing multiple links in a nav element to your .js file
I am very confused with the different methods used to select items from the DOM. I'm using 'document.getElementsTagName()' to get the links from the nav element. I'm not sure when to use 'document.querySelector()' or 'document.querySelectorAll()' in any case. Could someone please help me with the proper way of coding this solution. Thank you, Aaron
let navigationLinks = document.getElementsByTagName('nav');
let galleryLinks;
let footerImages;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Nick Pettit | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Nick Pettit</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="index.html" class="selected">Portfolio</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="img/numbers-01.jpg">
<img src="img/numbers-01.jpg" alt="">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="img/numbers-02.jpg">
<img src="img/numbers-02.jpg" alt="">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="http://twitter.com/nickrp"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="http://facebook.com/nickpettit"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© 2016 Nick Pettit.</p>
</footer>
</div>
<script src="js/app.js"></script>
</body>
</html>
1 Answer

Tim Knight
28,888 PointsHi Aaron,
I tend to stick with document.querySelector()
or document.querySelectorAll()
when I'm selecting elements personally. This is because I can do a much more complex selector than just selecting items by their tag name. This too lets you select elements within a parent element, just like CSS... for example nav a
. The difference between document.querySelector()
and document.querySelectorAll()
is really in the keyword "All". The first method will give you back the first item on the page were document.querySelectorAll()
will return all of the items on the page. So for your example within this first challenge, I would approach it like this:
let navigationLinks = document.querySelectorAll('nav a');