Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial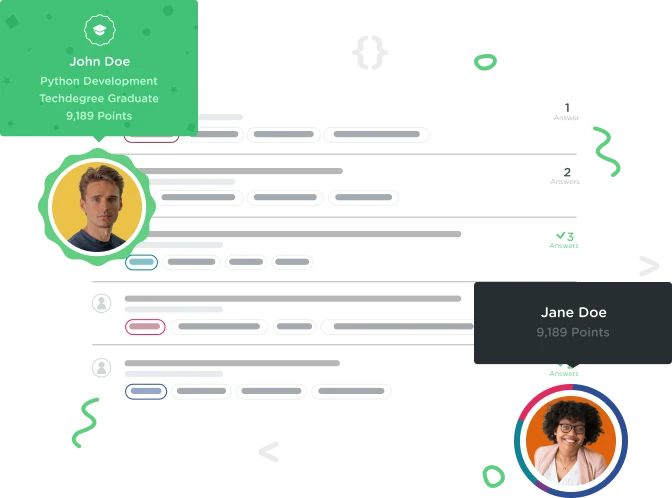

louiseretzlaff
8,325 PointsReferring a variable to a collection
What method would you use to give the item list variable the collection of the unordered list with the ID rainbow?
var listItems= document.getElementByTagName('ul') ;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
2 Answers

Tim Oltman
7,730 PointsHi Louise,
The function I think you're trying to use is getElementsByTagName(). There is no getElementByTagName (singular). This returns a collection of matching elements so you would need to access your child <li> elements with document.getElementsByTagName('ul')[0].children.
Alternatively, you could use document.querySelectorAll('ul#rainbow li') or document.getElementById('rainbow').children.
/* Any of the following selections work with your code */
// var listItems= document.getElementsByTagName('ul')[0].children;
// var listItems= document.querySelectorAll('ul#rainbow li');
var listItems= document.getElementById('rainbow').children;
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
A good way to check stuff like this is to open your html file in your browser and try to do the selections in the devtools console.

Zimri Leijen
11,835 PointsIf I understand correctly, you want to select the item by the id, and store it in a variable.
There's two ways to do that.
let variable = document.querySelector('#rainbow')
;
or
let variable = document.getElementById('rainbow')
;

louiseretzlaff
8,325 PointsYes, then I need to have the for loop going through the colors so each statement in the html doc is the correct color. I keep getting an error saying thereβs something undefined in the listItems[i].style