Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial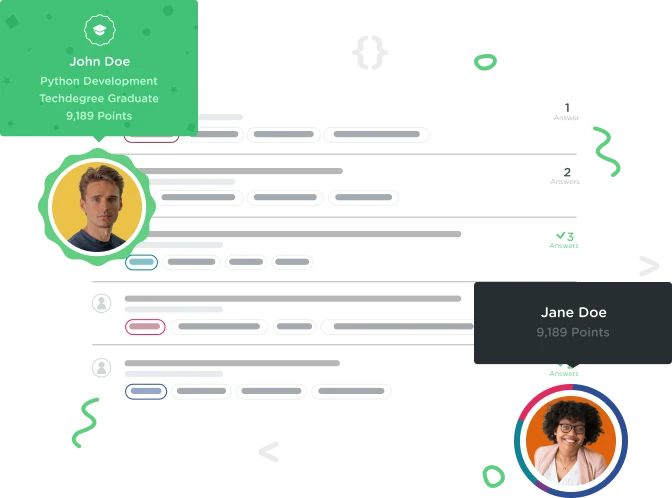
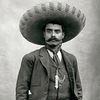
Keith Corona
9,553 PointsReferring to numbers and strings in an array
Hello all,
var answers = 0;
var wrong = 0;
var questions = [
['What is the lingua franca?', 'english'],
['What is the capital of California?', 'sacramento'],
['How many lives are cats rumored to have?', 'nine']
];
function print(message) {
document.write(message);
}
for (var i = 0; i < questions.length; i ++) {
var guess = prompt(questions[i][0]);
if (guess.toLowerCase() === questions[i][1]) {
answers += 1;
} else {
wrong += 1;
}
}
document.write('You answered correctly to ' + answers + ' question(s) and missed ' + wrong + ' question(s).');
My code, above, seems to work fine, but there is one problem. In my questions array, in the third question (index 2), I wanted to use the number 9 in place of the string nine. I remembered that before we had used parseInt, but with already having added toLowerCase, I wasn't sure how I could do this. What is the correct way to allow numbers and strings for our answers to quiz questions?
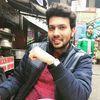
Abhishek Bhardwaj
3,316 Points.toLowerCase function only exists on strings. You can call toString() on anything in javascript to get a string representation.
1 Answer

Casey Ydenberg
15,622 PointsAccording to w3cshcools, the value returned from prompt is always a string. To ensure you're comparing strings to strings, use
['How many lives are cats rumoured to have?', '9']
Note the 9
is in quotation marks: still a string! You might want to test this across browsers, just to be sure the behaviour of prompt is consistent.
If you want to parseInt, you'll need a way to NOT apply to the other questions which ARE strings. This is more complicated - you'll need another if to determine whether the expected input can be considered as a number or not.
Abhishek Bhardwaj
3,316 PointsAbhishek Bhardwaj
3,316 Pointstry " toString() " Function.
For Example:
var ans = 334; var temp = ans.toString().toLowerCase(); alert(temp);