Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial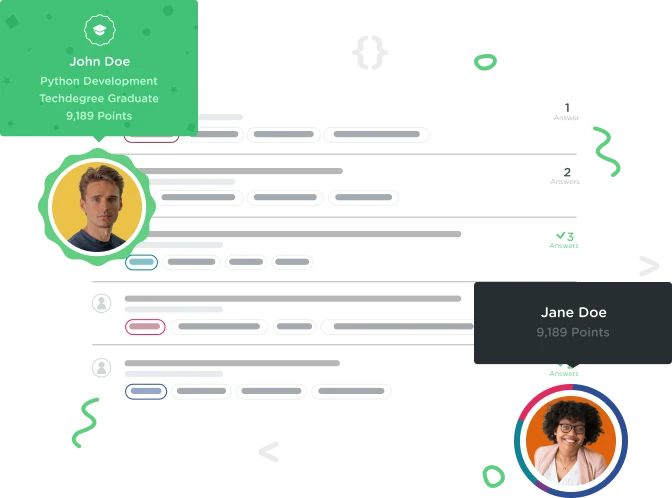
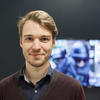
Lean Rasmussen
11,060 Pointsreferring to objects in arrays
I am having difficulties with referring to objects in arrays.
Normally when you make an object you assign it to a variable.
var name {
prop1 : value1,
prop2 : value2,
};
to refer to this I can just go name.prop1.
arrays we use position so eg. arrayName[0]
but if my object is inside the array it does not have a name. just a position.
array[{
prop1 : value1,
prop2 : value2,
},
2]
here I can refer to array[0] to get to the array, but if I wanna get to the properties I dont know how. I can still got array[0].prop1 but I am having diffculties going trough the properties of and object in an array.
3 Answers
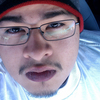
Chyno Deluxe
16,936 PointsHey Lean, I am assuming you are asking how to loop through an array of objects. So my answer is below as well as a demo.
First you need to create a for loop to cycle through your array. Then, within your for loop you need to create a for...in loop to cycle through your objects. Like this.
var myArr = [{
prop1: "Hello",
prop2: ", ",
prop3: "World",
prop4: "!"
},{
prop1: "Hello",
prop2: ", ",
prop3: "Internet",
prop4: "!"
}];
for (var i = 0; i < myArr.length; i += 1) {
for (var obj in myArr[i]) {
console.log(myArr[i][obj]);
}
}
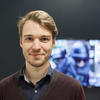
Lean Rasmussen
11,060 PointsAs you say this was in the demo. But what if I wanna see what properties are in the object inside my array. So that I display not just the values, but the properties also.
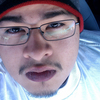
Chyno Deluxe
16,936 PointsYou can view all of the properties and values by logging your array to the console. Like this
for (var i = 0; i < myArr.length; i += 1) {
console.log(myArr[i]); //this will display all properties and values that are in your array
for (var obj in myArr[i]) {
result.innerText += myArr[i][obj];
}
}
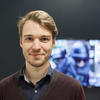
Lean Rasmussen
11,060 Pointsthank you for your help!!!