Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial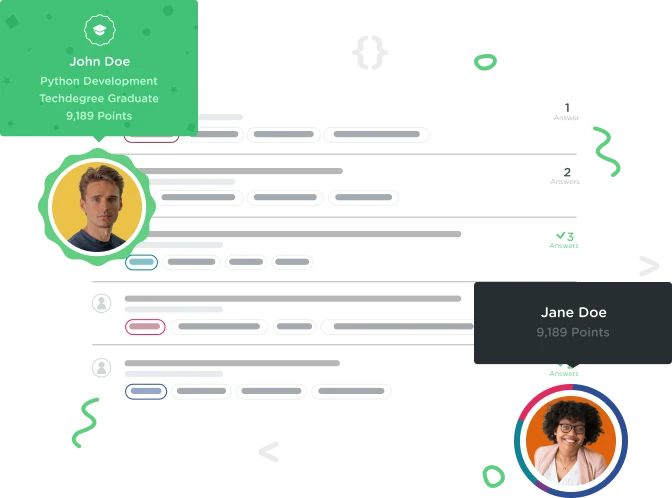

james white
78,399 PointsReflection Basics, Task 2 of 2
I have no idea what code to use for task 2 of 2...
Challenge Task 2 of 2
Code the getModifierString method below so that it returns a String representation of the given method's modifiers. For example, if a method has the signature public static void main(String[] args) then getModifierString would return "public static". Don't forget to add the import statement for the Modifier class.
Here is what I used to get past task 1 of 2:
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
public class ReflectionUtils {
// Task 1
public static Method[] getMethods(Class<?> clazz) {
// Get all declared methods
Method[] methods = clazz.getDeclaredMethods();
return methods;
}
// Task 2
public static String getModifierString(Method method) {
return null;
}
}
Note: the 'import java.lang.reflect.Modifier;' line is not needed for task 1 of 2,
but I think it will be needed for task 2 of 2.
5 Answers

james white
78,399 PointsI tried just copy paste of the above, but it didn't like the lines with 'm' in them.
So I slimmed it down to:
// Task 2
public static String getModifierString(Method method)
{
// Display modifiers
int mods = method.getModifiers();
String modStr = Modifier.toString(mods);
return modStr;
}
..which let me complete the course, and get 289 points.
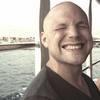
Martin Gallauner
10,808 PointsI know you posted this a long time ago...
the solution for the 2nd task is:
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
public class ReflectionUtils {
// Task 1
public static Method[] getMethods(Class<?> clazz) {
return clazz.getDeclaredMethods();
}
// Task 2
public static String getModifierString(Method method) {
int mods = method.getModifiers();
return Modifier.toString(mods);
}
}

james white
78,399 PointsOkay...so how to work that 'main.java' file code snippet above into something that
would make task 2 pass??
Hmmm...
Well the line that has 'methods' in it probably isn't going to pass
since we only have 'method' (singular).
So the line below it that has 'm.getModifiers();' isn't going to be kosher if
'm' is isn't defined in the line above it.
Alright, so we just get out our handy-dandy auto slider rule code adjuster
and out pops this code:
// Task 2
public static String getModifierString(Method method)
{
// Display modifiers
int mods = method.getModifiers();
String modStr = Modifier.toString(mods);
return modStr;
}
..and lo and behold that's all that is needed to pass!
Of course who really know what it does...
Maybe some code monkey can explain it to me someday,
but in the meantime I got my 289 points, as well as my Java Annotations badge,
so I'm calling it a day.

Ronny Kibet
47,409 PointsThanks for all this effort James. Got stuck for a while until I read your post. Cheers. -Ronny.

james white
78,399 PointsOkay I did a little research.
I searched specifically for this in Google:
"import java.lang.reflect.Modifier;" "getModifierString("
..and got 126 results..
One of the results was the code on this page:
https://searchcode.com/codesearch/view/22803721/
public static String getModifierString(int modifiers)
{
String result;
if (Modifier.isPublic(modifiers))
result = "public";
else if (Modifier.isProtected(modifiers))
result = "protected";
else if (Modifier.isPrivate(modifiers))
result = "private";
else
result = "";
if (Modifier.isStatic(modifiers))
result = "static " + result;
if (Modifier.isFinal(modifiers))
result = "final " + result;
if (Modifier.isNative(modifiers))
result = "native " + result;
if (Modifier.isSynchronized(modifiers))
result = "synchronized " + result;
if (Modifier.isTransient(modifiers))
result = "transient " + result;
return result;
}
..which I converted to this:
public static String getModifierString(Method method)
{
String result;
if (Modifier.isPublic(method))
result = "public";
else if (Modifier.isProtected(method))
result = "protected";
else if (Modifier.isPrivate(method))
result = "private";
else
result = "";
if (Modifier.isStatic(method))
result = "static " + result;
if (Modifier.isFinal(method))
result = "final " + result;
if (Modifier.isNative(method))
result = "native " + result;
if (Modifier.isSynchronized(method))
result = "synchronized " + result;
if (Modifier.isTransient(method))
result = "transient " + result;
return result;
}
This gave compile errors on all the if else lines, but not on the two lines that had the word 'result' in them.
So I tried this code:
// Task 2
public static String getModifierString(Method method)
{
String result;
result="None";
return result;
}
Which gave the message:
Bummer! Your method is not returning the correct String. Did you use the Modifier.toString(...) method?
Thus somehow 'Modifier.toString(' must be required.
You'll notice that the first code had 'int modifiers'.
So I'm thinking that this stupid .getModifierString call is designed to output some type of numeric code.
With a little more poking around the Java docs (<GASP> --those docs are sooooooo dry!)
I found this Class Modifier page:
https://docs.oracle.com/javase/8/docs/api/java/lang/reflect/Modifier.html
..which had these little tidbits:
Field Summary
Fields Modifier and Type Field and Description
static int ABSTRACT The int value representing the abstract modifier.
static int FINAL The int value representing the final modifier.
static int INTERFACE The int value representing the interface modifier.
static int NATIVE The int value representing the native modifier.
static int PRIVATE The int value representing the private modifier.
static int PROTECTED The int value representing the protected modifier.
static int PUBLIC The int value representing the public modifier.
static int STATIC The int value representing the static modifier.
static int STRICT The int value representing the strictfp modifier.
static int SYNCHRONIZED The int value representing the synchronized modifier.
static int TRANSIENT The int value representing the transient modifier.
static int VOLATILE The int value representing the volatile modifier.
Method Summary All MethodsStatic MethodsConcrete Methods Modifier and Type Method and Description
static int classModifiers() Return an int value OR-ing together the source language modifiers that can be applied to a class.
static int constructorModifiers() Return an int value OR-ing together the source language modifiers that can be applied to a constructor.
static int fieldModifiers() Return an int value OR-ing together the source language modifiers that can be applied to a field.
static int interfaceModifiers() Return an int value OR-ing together the source language modifiers that can be applied to an interface.
static boolean isAbstract(int mod) Return true if the integer argument includes the abstract modifier, false otherwise.
static boolean isFinal(int mod) Return true if the integer argument includes the final modifier, false otherwise.
static boolean isInterface(int mod) Return true if the integer argument includes the interface modifier, false otherwise.
static boolean isNative(int mod) Return true if the integer argument includes the native modifier, false otherwise.
static boolean isPrivate(int mod) Return true if the integer argument includes the private modifier, false otherwise.
static boolean isProtected(int mod) Return true if the integer argument includes the protected modifier, false otherwise.
static boolean isPublic(int mod) Return true if the integer argument includes the public modifier, false otherwise.
static boolean isStatic(int mod) Return true if the integer argument includes the static modifier, false otherwise.
static boolean isStrict(int mod) Return true if the integer argument includes the strictfp modifier, false otherwise.
static boolean isSynchronized(int mod) Return true if the integer argument includes the synchronized modifier, false otherwise.
static boolean isTransient(int mod) Return true if the integer argument includes the transient modifier, false otherwise.
static boolean isVolatile(int mod) Return true if the integer argument includes the volatile modifier, false otherwise.
static int methodModifiers() Return an int value OR-ing together the source language modifiers that can be applied to a method.
static int parameterModifiers() Return an int value OR-ing together the source language modifiers that can be applied to a parameter.
static String toString(int mod) Return a string describing the access modifier flags in the specified modifier.
Hey! Are we having fun yet!
All that garbage from the java docs is meant to convey that there is a table whereby
specific numerical (int) values have a string equivalent (I think).
Also tried:
// Task 2
public static String getModifierString(Method method)
{
String result;
result= method.Modifier.toString();
return result;
}
..which gave the same Bummer message:
Bummer! Your method is not returning the correct String. Did you use the Modifier.toString(...) method?
.
...but gave no compiler errors in output.html.

james white
78,399 PointsI was tempted to look at the "Introduction to Reflection" course video again,
but without an .srt transcript file it was useless.
So I took at look at the S2V6 project zip download, focusing on the 'main.java'
file inside the '\src\com\teamtreehouse\docgen' sub folder.
It had the following code:
public static void main(String[] args) {
// Get a class object
Class<?> clazz = MathUtils.class;
// Get all declared methods
Method[] methods = clazz.getDeclaredMethods();
// Loop over methods
for(Method m : methods) {
// Display modifiers
int mods = m.getModifiers();
String modStr = Modifier.toString(mods);
System.out.printf("\tmodifiers: %s%n",modStr);
}
This code snippet included the mysterious 'Modifier.toString(' call
so it might be relevant/significant..