Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial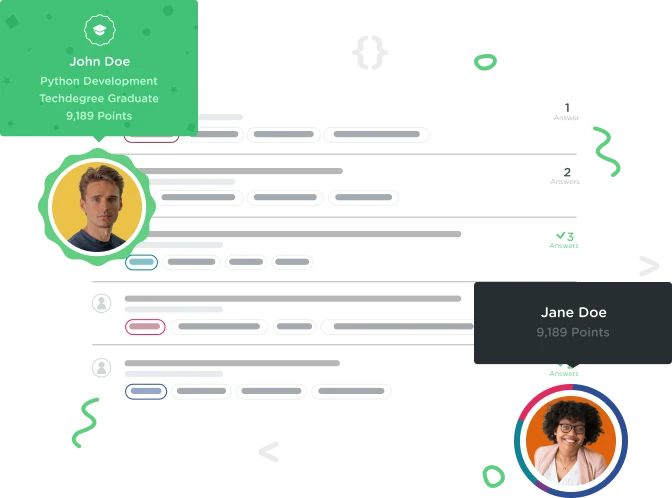
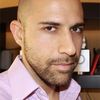
Imer Pacheco
9,732 PointsRegard the "score" method in the game.py, in the Object-Oriented Python course.
I'm getting a syntax error in the section that tries to add 1 to the self.current_score section. To me it looks correct but apparently it is not. Any guidance on this matter would be greatly appreciated.
Here is my code:
class Game:
def __init__(self):
self.current_score = [0, 0]
def score(player):
if player == 1:
self.current_score[0] = self.current_score[0] += 1
else:
self.current_score[1] = self.current_score[1] += 1
4 Answers
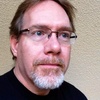
Chris Freeman
Treehouse Moderator 68,454 Pointsyou can not mix multiple variable assignments with the "plus equals" incremental assignment
# change
self.current_score[0] = self.current_score[0] += 1
# to either
self.current_score[0] = self.current_score[0] + 1
# or to
self.current_score[0] += 1
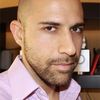
Imer Pacheco
9,732 PointsAh yes! I was thinking to myself "How is the value of the player argument being relayed to the self.current_score attribute? Now I know. Thank you again Mr. Freeman!
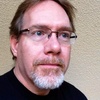
Chris Freeman
Treehouse Moderator 68,454 PointsMy pleasure and please... call me Chris
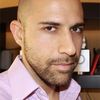
Imer Pacheco
9,732 PointsYou got it, Chris!

Jonathon Mohon
3,165 PointsWill all methods of a class require the self parameter because I made this exact mistake myself.
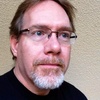
Chris Freeman
Treehouse Moderator 68,454 PointsJonathon, All methods of a class should have a first parameter to represent the class instance referred to (usually "self
" for instance methods), or the the class itself (usually "cls
" for class-based methods). Note the actual variable names can be anything "self" and "cls" are simply conventions for readability.
Imer Pacheco
9,732 PointsImer Pacheco
9,732 PointsHello again, Mr. Freeman! Thanks again for your input, however, I am still struggling with this challenge. Here is my revised code:
I'm sure it's something silly but I can't seem to put my finger on it. Thanks!
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsApologies. I should have caught this error on initial inspection. The method
score()
must have the instance as the first parameter:def score(self, player): # <-- added 'self'
James N
17,864 PointsJames N
17,864 Pointsthanks! this also helped me out! (i couldn't get past a few codeChallenges because of the self attribute...)