Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial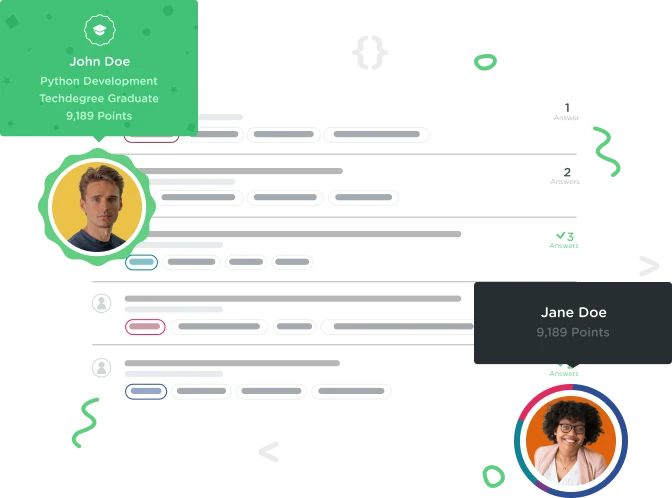

Ra Bha
2,201 PointsRegarding 2:59 - 3:45, can anybody provide a fuller explanation on why Jeremy is taking the steps he is taking?
During this time, Jeremy states "We'll make it private readonly. Then in the constructor we'll set it to the path that was passed in, and I'll change this to use the field instead. Now we can go back to the move method and use the object's instance of the path to update the location property to the invader's new location. "
But why is he doing this. Can anybody provide a step by step explanation on why he is taking each of these steps? In what situations would the use of an object's instance be warranted rather than a new property with a getter and setter?
1 Answer

Paema Hare
22,705 PointsI'll make an attempt at answering this.
Inside the Invader class, he created the field '_path' to store the location of the path that is on the map, so that the invaders created know where they are supposed to go. Since the path should not ever get changed by either the Invaders themselves, or any external use of the class, the field that holds the information on the path is set to private and readonly; we just want to see where the path is.
Inside the constructor for the Invader class, we need to save the path location to the field '_path', so you end up with:
_path = path;
Now, inside the constructor we already have:
Location = path.GetLocationAt(_pathStep);
We change it to read:
Location = _path.GetLocationAt(_pathStep);
I believe he makes use of the '_path' field here for a kind of consistency. Only use the original 'path' that was passed in to the constructor once, then use the field '_path' that was set, because '_path' exists for everything else inside the class to use.
That part is a bit harder for me to make clear tbh. I assume it is a way for us to get used to using the fields too.
Now, the why we are making this '_path' field instead of a getter and setter: We are only going to set the '_path' field once, and we will be doing it on the initialization of a new invader. I think the getter and setter is used more when you need to get and set multiple times. Also, the getter/setter is used to allow you to NOT access a field directly, keeping the field safe from accidental changes. Since '_path' is private readonly, it is safe to use it directly.
Now inside the method 'Move()' we have already updated '_pathStep' by adding 1 to it. Next we need update the actual Location information for the Invader. That is:
Location = _path.GetLocationAt(_pathStep);
We use field '_path' because that is the only way this method knows where the map's path is. Then we pass in the updated '_pathStep', since the Invader has moved, so that the 'Location' can know the coordinates of the new position on the path.
I think this is where the consistency comes in with the above constructor method. We do the exact same thing in the method 'Move()' as we did in the constructor. This probably provides clarity of what is happening in both methods.
Hopefully this explanation is helpful enough.