Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial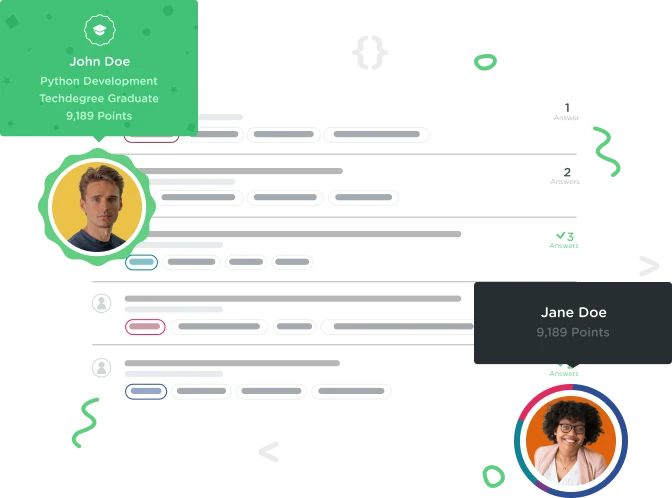

Nir Klein
7,353 PointsRegarding 'Equals' override
Hi there, I've been working on the following challenge: https://teamtreehouse.com/library/intermediate-c/systemobject/objectequals
The challenge is about overriding 'Equals' in 'VocabularyWord' class.
Get someone share a hint about what I might missing here? (attached my code)
Thanks!
using System;
namespace Treehouse.CodeChallenges
{
public class VocabularyWord
{
public string Word { get; private set; }
public VocabularyWord(string word)
{
Word = word;
}
public override string ToString()
{
return Word;
}
public override bool Equals(object obj)
{
if(obj != null)
{
string checkThat = obj as string;
if(Word.Length != checkThat.Length)
{
return false;
}
for(int i = 0; i < Word.Length; i++)
{
if(Word[i] != checkThat[i])
{
return false;
}
}
return true;
}
else
{
return false;
}
}
}
}
2 Answers
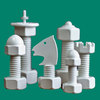
Steven Parker
241,783 PointsYou're close! But the objects being compared are both VocabularyWord objects, so it doesn't make sense to cast one as a string. Instead, you can cast it to a VocabularyWord and then access the Word inside it:
string checkThat = (obj as VocabularyWord).Word;
Once you've done that, the rest of the code will work, but you really only need to compare the strings directly:
return Word == checkThat;

Nir Klein
7,353 PointsIt works! thanks!