Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial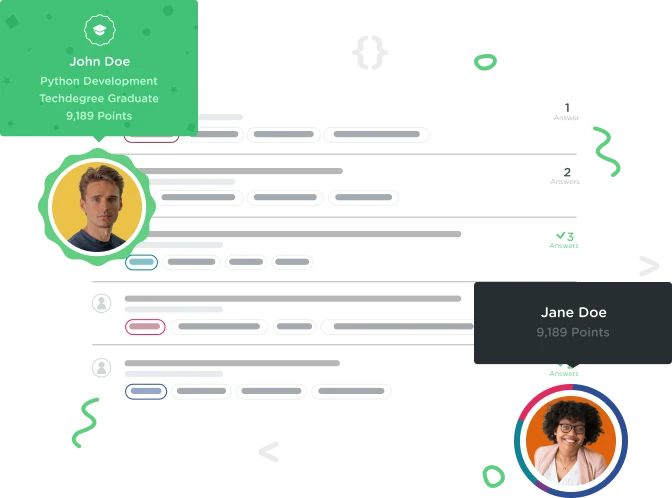

Louis Darby
5,149 PointsRegarding Prototypes
Hi, I was wondering if it is possible to write a function that essentially searches through all of one category of your prototype object. Say you made lots of new Person objects with your prototype, could you then retrieve all objects with one property. For example, could you write an if statement that said if age = x print name? Essentially searching through the data you've made via new prototype.
2 Answers

Kevin Lozandier
Courses Plus Student 53,747 PointsHi, Lous:
Yes, JS allows you to check for a particular property through hasOwnProperty
which you can use to do what you seek to accomplish.
Perhaps even better for your case is to set the property's enumerable
value to false
in order for it to automatically be ignored in any enumerable operation you do throughout your program.
Does this make sense?
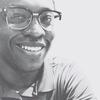
Ricardo Hill-Henry
38,442 PointsI'm not sure if I quite understand, but it sounds certainly do-able. You'd just make an array, populate it with your various Person instances, loop through the array setting x equal to the index and loop through something similar to:
//Assume personsArray has been populated with multiple Person prototypes
for(int x = 0; x < personsArray.length; x++){
if(personArray[x].age == 18){
print(personsArray[x].name);
}
}
//Would be better to just set a variable to represent personsArray[x] to follow DRY
Very sloppy code, but just look at it as a basis. I'll review it when I'm not busy.

Louis Darby
5,149 PointsThanks for the response. My fault, i'll try and clarify a bit. If you had your prototype set up as was shown (correct me if I'm wrong):
var personPrototype = {
name: "anon",
age: "number",
place_of_birth: "location",
function Person (name, age, place_of_birth) {
this.name = name,
this.age = age,
this.place_of_birth = location
Person.prototype = personPrototype
and then you made your new objects using the prototype e.g;
Louis.new Person ("Louis",24,"London");
John.new Person ("John",25,"Madrid");
Chris.new Person ("Chris",22, London");
Could you then create a function, such as an if statement that said if the place_of_birth = "London", console.log (name). But would still work no matter how many objects you made through the prototype?
Hope that makes more sense, appreciate the help.
Louis Darby
5,149 PointsLouis Darby
5,149 PointsHi,
Thanks for the help! It does make sense! However, I'm trying to check for a value of the property rather than if an object has a particular property. Is that possible to do this? For example as mentioned before, all objects will share the place_of_birth property but will vary in their values, I want to be able to see which objects have a value of London for their place_of_birth property.
Appreciate the help.
Kevin Lozandier
Courses Plus Student 53,747 PointsKevin Lozandier
Courses Plus Student 53,747 PointsHello again, Louis Darby:
You can in fact do this after you verified that the object has the property you want to check the value of; you simply add an additional
if
/else
clause or aternary
operator (?
) to accomplish this.