Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial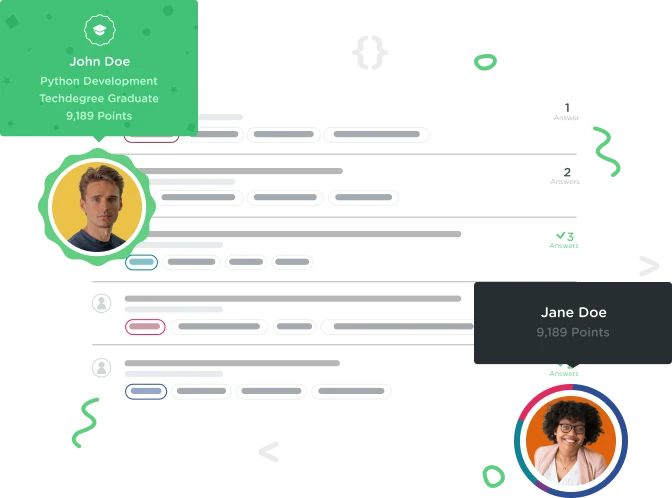
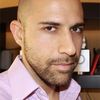
Imer Pacheco
9,732 PointsRegarding the "combo" function in the Python Collections course.
In Workspaces my python code is only returning the last part of the list of tuples I need. According to the challenge, I should be getting the following list of tuples: [(1, 'a'), (2, 'b'), (3, 'c')] however, I'm only getting: [(3, 'c')] I'm sure what I am doing wrong is something silly but I can't seem to put my finger on it. Any guidance would be greatly appreciated. Thanks!
NOTE: The code below is written so that Workspaces prints a result in the console and not for returning a value like it asks for in the challenge.
list1 = [1, 2, 3]
list2 = 'abc'
def combo(itr1, itr2):
tup_list = list()
for num in itr1:
a = num
for letter in itr2:
b = letter
new_tup = a, b
tup_list.append(new_tup)
print(tup_list)
combo(list1, list2)
3 Answers
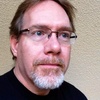
Chris Freeman
Treehouse Moderator 68,454 PointsHi Imer, Your two for
loops each run to completion with the final values left in the variables a
and b
.
You need to place the append
assignment inside the for loops so that a new tuple is added each iteration.
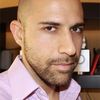
Imer Pacheco
9,732 PointsMr. Freeman! Good to hear from you again. Thank you for the different variations of the "combo" challenge code. I used the first version to pass the challenge. The other variations look interesting but I did not feel comfortable using themsince I have yet to learn about the zip() method. However, I will definitely look into it now that you have brought it to my attention. Thanks again!
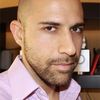
Imer Pacheco
9,732 PointsOh wow! Only one line of code after defining the function? Impressive. I can't wait to be able to write code like that. Thanks again, Mr. Freeman!
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsThere are a couple of ways to do this:
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsI found one more variation using list comprehensions you'll get to later on: