Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial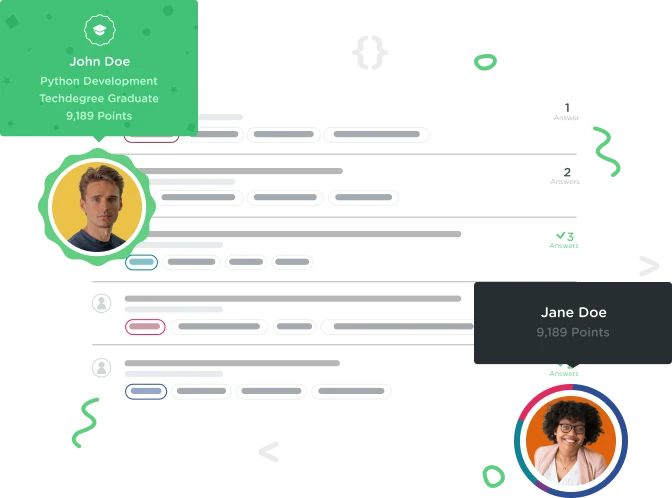
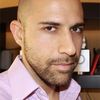
Imer Pacheco
9,732 PointsRegarding "Word Count" challenge in Python Collections lesson
My code evaluates correctly in Workspaces but it does not pass the "Word Count" challenge in the Python Collections lesson. I am not clear on what I am doing wrong. Any guidance would be much appreciated. Thanks!
my_string = "I am that I am"
def word_count(a_string):
low_str = a_string.lower() # This converts all all letters to lower case
spl_str = low_str.split() # This splits each word into a list
my_dict = {}
a_list = []
b_list = []
c_list = []
for word in spl_str:
if word == spl_str[0]:
a_list.append(word)
my_dict.update({word: len(a_list)})
continue
elif word == spl_str[1]:
b_list.append(word)
my_dict.update({word: len(b_list)})
continue
elif word == spl_str[2]:
c_list.append(word)
my_dict.update({word: len(c_list)})
continue
else:
break
print(my_dict)
return(my_dict)
word_count(my_string)
my_string = "I am that I am"
def word_count(a_string):
low_str = a_string.lower() # This converts all all letters to lower case
spl_str = low_str.split() # This splits each word into a list
my_dict = {}
a_list = []
b_list = []
c_list = []
for word in spl_str:
if word == spl_str[0]:
a_list.append(word)
my_dict.update({word: len(a_list)})
continue
elif word == spl_str[1]:
b_list.append(word)
my_dict.update({word: len(b_list)})
continue
elif word == spl_str[2]:
c_list.append(word)
my_dict.update({word: len(c_list)})
continue
else:
break
print(my_dict)
return(my_dict)
word_count(my_string)
2 Answers
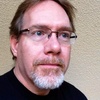
Chris Freeman
Treehouse Moderator 68,423 PointsYour code can only handle strings of words where all words march one of the first three words in the string. It would fail if the input string were "I I am am that" and also fails for when there are more than three different words "I am all that I am".
Can you think of a solution that loops through the words and adds them to a dictionary: word_counts[this_word] += 1
?
You will need to check if the word hasn't been seen before: if this_word not in word_counts:
Post back if you need more hints. Good Luck!
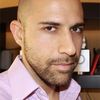
Imer Pacheco
9,732 PointsThe code is so much cleaner and readable. Thanks Chris! I truly appreciate your input in my python education. I hope to communicate with you more in the future. Here is my revised code:
def word_count(a_string):
words = a_string.lower().split() # Converts all letters to lower case then splits each word into a list
my_dict = {}
for word in words:
if word not in my_dict:
my_dict[word] = 1
else:
my_dict[word] += 1
return(my_dict)
Imer Pacheco
9,732 PointsImer Pacheco
9,732 PointsMr. Freeman, Thank you very much! After taking your guidance into consideration I was able to formulate a better solution and, ultimately, pass the challenge!
Here is my code:
I appreciate the time you took to answer my question, Mr. Freeman. :)
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsCongratulations on completing the challenge. If I could provide feedback on your solution. Once a solution is reached the code can be reviewed for simplification opportunities.
word_count
should end with areturn
statement instead of a print statementif
andelif
are complementary events (the word either is or is not in my_dict), the finalelse / break
will never happen and can be removedelif
can be replaced byelse
value
is reset each loop and only used asvalue + 1
, thevalue + 1
can be replaced by1
continue
at the end. This makes thecontinue
statements in theif
statement unnecessary and they can be removed.Here is your code simplified:
Additionally, the first two statements could be combined, if so desired:
words = a_string.lower().split()
The
dict.update
can be replaced with an assignment:`
my_dict[word] = 1
Keep up the good work!
-- Chris