Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial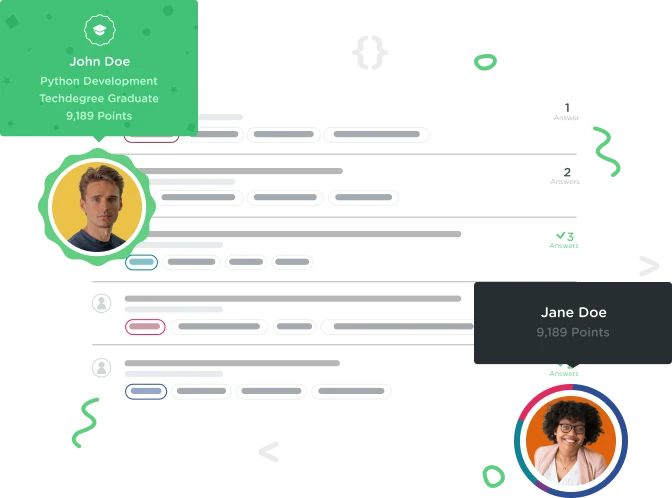
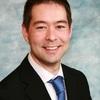
Mark Chesney
11,747 Pointsregex players dictionary and class
Hi. Can someone please help? The code below only shows the first entry, not all of them. Thanks in advance!
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search(r"""
^(?P<last_name>[\w ]*),[\s]
(?P<first_name>[\w ]*):[\s]
(?P<score>[\d]{2})$
""", string, re.X | re.M)
print(players.group())
print(players.groupdict())
4 Answers
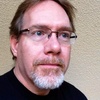
Chris Freeman
Treehouse Moderator 68,423 PointsYou are on the right track. re.search
only returns the first match. Perhaps you meant to use re.findall
which returns a list of matches:
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.findall(r"""
^(?P<last_name>[\w ]*),[\s]
(?P<first_name>[\w ]*):[\s]
(?P<score>[\d]{2})$
""", string, re.X | re.M)
print(players)
# [(‘Love’, ‘Kenneth’, ‘20’), (‘Chalkley’, ‘Andrew’, ‘25’), (‘McFarland’, ‘Dave’, ‘10’), (‘Kesten’, ‘Joy’, ‘22’), (‘Stewart Pinchback’, ‘Pinckney Benton’, ‘18’)]
Post back if you need more help. Good luck!!!
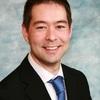
Mark Chesney
11,747 PointsThanks Chris! Okay, what you're saying making sense. Now can we get all the players into a grouped dict-like expression? Your code returns all players but ungrouped :|
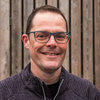
Chris Guy
Python Web Development Techdegree Graduate 14,615 PointsHi all,
Following on from Mark Chesney 's question above, I am stuck on how to iterate through matches to create instances of a class. Specifically I am struggling with this code challenge:
Wow! OK, now, create a class named Player that has those same three attributes, last_name, first_name, and score. I should be able to set them through init.
I have changed the regex to use re.finditer and added a class with an init function. When I run this in the console I can create an instance of the Player class which contains the player info from the final iteration of the loop.
So my questions are
- How would I go about creating a separate instance of the class for each of the players?
- How do i pass this specific challenge as the code below gives a Bummer:
players
doesn't seem to be a regex object. error.
Thanks in advance for any help.
Chris
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.finditer(r'''
^(?P<first_name>[\w]+\s?[\w]+?)
,\s(?P<last_name>[\w]+\s?[\w]+?)
:\s(?P<score>[\d]+)$
''', string, re.X | re.MULTILINE)
class Player:
"""Define the Player class."""
def __init__(self, last_name=None, first_name=None, score=None):
"""Customize the class initiation."""
for match in players:
self.first_name = match.group('first_name')
self.last_name = match.group('last_name')
self.score = match.group('score')
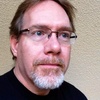
Chris Freeman
Treehouse Moderator 68,423 PointsChris Guy, Don’t let the extra work Mark and I did confuse you. We went beyond the challenge to explore other capabilities of python.
You do not need to use finditer
. You can stay with self
or match
. The re
expression does not need to change in Task 2.
In the Player
class, a loop is not needed. You can simply assign the attributes with the values passed in to the __init__
method. there is no need to reference the regex.
Post back if you need more help. Good luck!!!
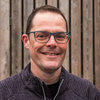
Chris Guy
Python Web Development Techdegree Graduate 14,615 PointsHi Chris Freeman - thank you, this makes sense now!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThere is also re.finditer which produces an iterator: