Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial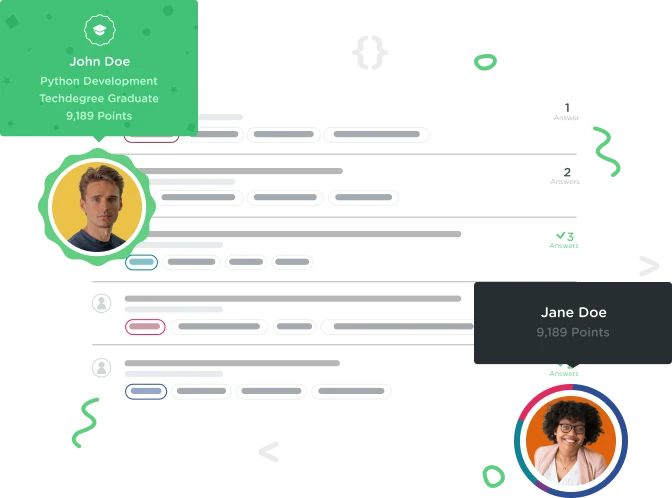
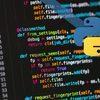
Bijay Gurung
1,818 Pointsregular expression
Create a function named find_words that takes a count and a string. Return a list of all of the words in the string that are count word characters long or longer. my solution: def find_words(count,string): re.findall(r'\w{count,}', string)
But this does not work, why?
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(count,string):
re.findall(r'\w{count,}', string)
4 Answers
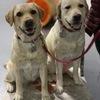
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Bijay,
You are finding the matches but then you are doing nothing with them. You need to return
the matches.
Also, your pattern isn't working.Python doesn't know to use the value from count
in your raw string. One way around this is to use string concatenation to build up a string with count in it (i.e., start the string with everything before count, then end the string, then concatenate count (after converting it to a string) then concatenate the rest of the string).
Hope that helps
Alex

Julie Luisi
1,782 PointsI am having the same issue, though I did put return in front of the re.findall(). The rest of my code is like Bijay's. I'm not quite following Alex's instructions on concatenating. A little more help please. Thanks a bunch! Julie
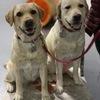
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Julie,
Suppose you are building a regex that needs to match a certain number of occurrences of a particular character. And you don't know ahead of time how many occurrences it is going to be. Let's further suppose that somewhere else in the code is handling getting this number of occurrences and putting it in the variable occurrences
.
Let's imagine that the character we were looking to find repeated was a
.
First, let's remind ourselves what this would look like if we could just write the number of occurrences straight into the regex, in this example we want to find matches of exactly 4 times:
pattern = r'a{4}'
So far, so easy. Now instead of 4
, we want the variable occurrences
. What we can't do is inject a variable into the string, so instead we use string concatenation.
Quick refresher on string concatenation:
concatenated_string = 'hello ' + 'world'
We're using the +` operator to join two string literals together. We don't have to just use string literals though, we can also use variables:
name = 'Princess Bubblegum'
concatenated_string = 'hello ' + name
We can use this principle to get our occurrences
variable into our regex pattern:
pattern = r'a{' + str(occurrences) + '}'
(Note that we've turned occurrences
into a string because all the values being concatenated must be strings, but occurrences
was an int).
Hope that was clear.
Cheers
Alex

Aaron Chi
173 PointsHello I am still a bit confused.