Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial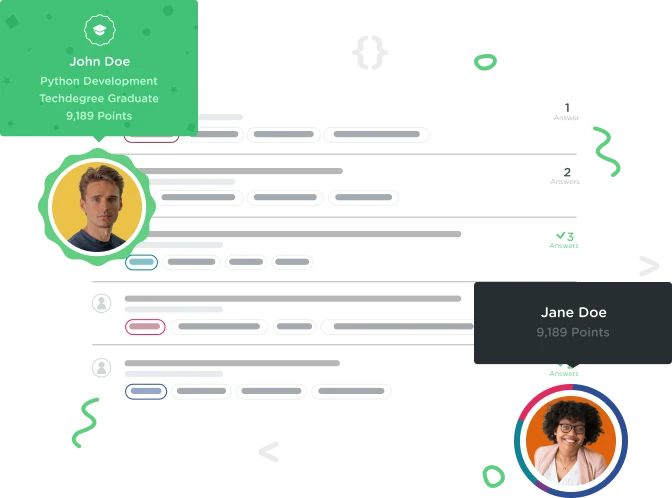

Josh Keenan
19,652 PointsRegular expressions uses
Just started the course and I was wondering whether they can be used in databases and variables like dictionaries, lists or tuples. Thanks Guys!
4 Answers
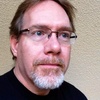
Chris Freeman
Treehouse Moderator 68,423 PointsIf by "can be used it" you mean stored within, then yes, to some degree. In lists and dictionaries, regex can be held like any other object.
In [1]: import re
In [2]: pat1 = re.compile(r'\w+')
In [3]: pat2 = re.compile(r'\d+')
In [4]: pats = [pat1, pat2]
In [6]: s1 = "this is a stirng with 01234 and ?##***882"
In [9]: pats[0]
Out[9]: re.compile(r'\w+')
In [11]: pats[0].findall(s1)
Out[11]: ['this', 'is', 'a', 'stirng', 'with', '01234', 'and', '882']
In [12]: pats[1].findall(s1)
Out[12]: ['01234', '882']
In [13]: pat_dict = { 'words': pat1, 'numbers': pat2 }
In [15]: for name, pattern in pat_dict.items():
print("{} in s1 are {}".format(name, pattern.findall(s1)))
....:
numbers in s1 are ['01234', '882']
words in s1 are ['this', 'is', 'a', 'stirng', 'with', '01234', 'and', '882']
A regexp can be stored in databases if properly serialized to match the limitations of the database format. It can also be stored by using the pattern string as it's value, then recreated from it stored value when needed.
In [19]: pat_dict['words'].pattern
Out[19]: '\\w+'

Iain Simmons
Treehouse Moderator 32,305 PointsGood answers here already, but if you're wondering if you can do a 'find and replace' sort of action within a list, dict, tuple, or external database, then it's more a case of 'sort of'... Regex is really only used on strings or text, wherever that text may reside.
So you could, for example, get a list of the key names in a dict, and then iterate/loop over them and use regex on each one to determine if it matches your expression.
You can't however, just apply a regex function to an entire list/dict/tuple.
As for a database, it depends on which type of database you're using, but some support selecting rows matching a regular expression:
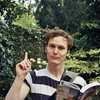
Bart Bruneel
27,212 PointsHey Josh,
Most people use regular expressions to search for (and maybe) replace specific things in large text-files and in writing web scrapers (there is currently a good course going on on Coursera about python and web scrapers if you are interested). I think you can also use regex to query databases. In Django, simple regexes are used to write views. Of course regexes are compatible with lists, dictionaries and tuples. For example, the re.findall method will return a list.

Josh Keenan
19,652 PointsChris and Bart, can either of you help with this? https://teamtreehouse.com/community/attributeerror-anonymoususermixin-object-has-no-attribute-username-2