Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial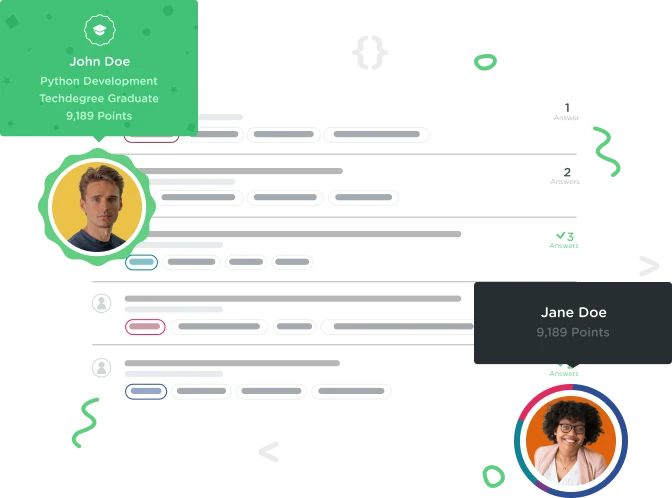
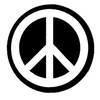
john larson
16,594 PointsReiterate when to use () when referring to a function, do I have it right?
Tell me if I got this right about when to use () when referring to a function: if we want to call the function right then, use (), if we just want to get it's value, just use the function name.
3 Answers
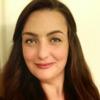
Jennifer Nordell
Treehouse TeacherBut... they do have parentheses! Now, if I'm not totally mistaken: the
passwordEvent
, confirmEvent
, and enableSubmitEvent
are being passed in as objects. They're events which are objects and may contain in themselves methods.
Both .focus
and .keyup
are jQuery methods. This is what we refer to as method chaining. Keep in mind that "method" is a fancy name for a function that happens inside an object's definition. And the things inside the parentheses are arguments that are being sent to the methods. So we're sending the event objects into the .keyup
and .focus
functions/methods.
Here's the documentation for .keyup
:
And .focus
:
You can have a function assigned to a variable and send in the variable name. But other than that, I'm still not sure I know of any instance where we call a function without the parentheses. But if I'm wrong, please someone tell me!
edited for accuracy
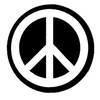
john larson
16,594 Points//here is the function passwordEvent:
function passwordEvent(){
if(isPasswordValid()){ /*condition references fn above */
$password.next().hide(500);
} else {
$password.next().show(500);
}
}
//this is where the function is used
$password.focus(passwordEvent)
//so focus is the method and
//passwordEvent (a function) is passed into the method,
// but there are no ().
//I would think(I know it isn't so, but trying to understand)
//why it doesn't look like this
$password.focus(passwordEvent())
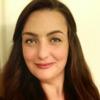
Jennifer Nordell
Treehouse TeacherAh ha! I see what you mean. I was confused by the "get its value" part of your question. Here, we're calling the .focus
method and we're sending in the "function expression" as an argument. Which is somewhat similar to what I stated about assigning a function to a variable name.
But in reality, we're not calling or executing that function. The .focus
method is calling/executing that function.
What I thought you were referring to is something like this:
function addTwo() {
return 2 + 2;
}
var x = addTwo();
console.log(x);
In which case the it has a value returned (although useless) And here if we left off the parentheses it wouldn't work really. The value of x would be assigned the entirety of the addTwo function. In short, if you're sending in a named function expression as an argument to another function then, no, you don't need the parentheses
edited for additional note
Incidentally, every function in JavaScript is an object. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Function
So when you send it in this way, you are sending in the entirety of the Function object.
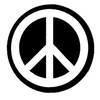
john larson
16,594 Points"But in reality, we're not calling or executing that function. The .focus method is calling/executing that function."
That line helps it make sense to me, Thank you. Maybe I could say it this way. If a method has a function passed to it, don't add parenthesis?
Thanks again Jenifer.
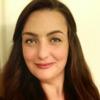
Jennifer Nordell
Treehouse TeacherIn this case, no. Because we're sending in the entirety of the function object as an argument
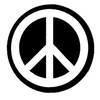
john larson
16,594 PointsFantastic! So now I know enough to know what my actual question is: ..."Because we're sending in the entirety of the function object as an argument"... In what situations will I send in the entirety of the function object as an argument?
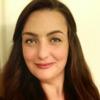
Jennifer Nordell
Treehouse TeacherShort answer? The only case I've seen it used in is when defining events . Now, there may be other times, but I haven't found them yet
By the way, if you take a peek again at the .focus
documentation, you'll see that it accepts a Function( Event eventObject )
which is an indicator that it takes the entirety of the function object. Not just the results/calling of the function.
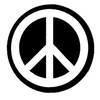
john larson
16,594 Points..."if you're sending in a named function expression as an argument to another function then, no, you don't need the parentheses"...
Just to clarify, (again, sorry and thank you for your time): Applying your statement to this scenario...
$password.focus(passwordEvent):... focus is the function [or the method]... passing in a function (passwordEvent)...leave off the parenthesis.
Just so you know, I'm not lazy...but when I look up things on MDN, I just don't get it. ie: that objectObject thing you mentioned. You said it represents the ENTIRETY of the object. That's important. I didn't know that. Even if I did I wouldn't have known the implications, but now that you said it I have a better idea. Thanks :D

Kristian Gausel
14,661 PointsIf by value you mean a reference to the function, which you can call later then it is correct.
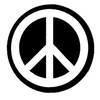
john larson
16,594 PointsI didn't give enough details to ask the question properly. In the code below: passwordEvent, confirmEvent and enableSubmitEvent; are all functions referenced here without using (). It has been confusing to me when to do so. I was just checking if I had codified the concept into a cohesive thought. I see the points you made and I guess the answer is ... I'm closer.
$password.focus(passwordEvent).keyup(passwordEvent)
.keyup(confirmEvent).keyup(enableSubmitEvent);
$confirmPassord.focus(confirmEvent).keyup(confirmEvent).keyup(enableSubmitEvent);

Kristian Gausel
14,661 PointsThis is because in this case you pass a reference to the function, not the result of executing the function.
function example (callme){
callme("This will print");
}
example(function(whattoprint) {console.log(whattoprint);});
I think this example could clarify a little
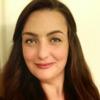
Jennifer Nordell
Treehouse TeacherKristian Gausel is correct. We are passing the function object, but we don't need a value back from the function. Had we needed a value back from the function to be passed into the function calling it then we would have issued a call to the function as denoted by parentheses. Otherwise we're passing the function object.
And just to further clarify Kristian's point, here's an example of two that are dependent on us getting back a value from the call to the function:
function addUs() {
return 7 + 5;
}
function squareUs(num3) {
return num3 * num3;
}
var result = squareUs(addUs());
console.log(result)
john larson
16,594 Pointsjohn larson
16,594 PointsKristian and Jennifer, Thanks to both of you...very insightful.