Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial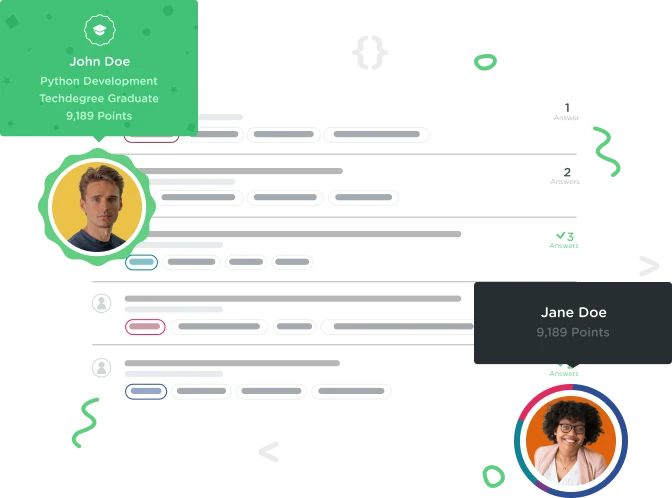
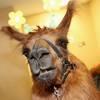
A X
12,842 PointsRemember Method Chaining? I sure don't :(
Craig mentions in the first video of the Objects part of the Java course: "remember method chaining?" And then strings together a bunch of commands (methods?) using dot notation..but he only I believe used method chaining with && in the compiler before...so what is method chaining? Is it simply chaining together a bunch of parts of your program to run at once like toLower.firstName.equals? If so, in what instances do we use method chaining? Thanks for clarifying!
P.S. What video does he mention method chaining? I don't even recall that topic...
3 Answers
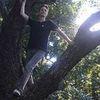
gyorgyandorka
13,811 PointsFirst, using boolean operators (&&, ||) in the shell to combine the results of commands is a different thing.
Methods are functions bound to an object/type, and are called (in Java and similar languages) by this dot notation syntax object.method()
.
In this example below, myName
is an object of type String
(i.e., an instance of the String
class). The String
class has this certain method, toLowerCase()
defined in it, which is a function that returns another string object with the same letters as the string on which the method was called, but all of them lowercased.
Here comes the trick: since the toLowerCase()
method returns a string, and the expression myName.toLowerCase()
thus evaluates to a new String
type object, you can call some other method (available for String
objects) directly on this expression, without storing its value in a new variable. You can use this method chaining syntax in many languages.
String myName = "George";
String myNameUpper = myName.toLowerCase().toUpperCase(); // returns "GEORGE"
gives exactly the same result as:
String myName = "George";
String myNameLower = myName.toLowerCase();
String myNameUpper = myNameLower.toUpperCase(); // returns "GEORGE"
In the first case we just skipped a step, and didn't store the return value in a separate variable - instead we called the toUpperCase()
method directly on that String
object which is returned by myName.toLowerCase()
.
You can chain as many methods as you like, as long as the actual method can be called on the type returned in the previous step:
String myName = "Adam";
String myNameLower = myName.toLowerCase().toUpperCase().toLowerCase(); // returns "adam"
// ( == "adam" ) == "ADAM" ) == "adam" )
Another method chaining example:
boolean containsZero(int myInt) {
// returns whether the integer given as argument contains a 0 digit
return String.valueOf(myInt).contains("0");
}
The valueOf()
method (defined inside the String
class) returns a string representation of an integer passed in as argument. So the expression String.valueOf(1013)
evaluates to the String
object "1013"
, and now you can call any String
methods on it (like contains()
, which returns true
if the string contains the sequence of characters passed in as argument).
The following is not strictly relevant to the answer, if it is too advanced/dense/confusing for the moment, feel free to skip this. If we'd like to be extremely clear, then object.method()
above could be written as variableOfTypeX.methodAvailableForTypeXEntities()
. A class is one kind of a type, but e.g. interfaces are also types with methods associated with them - the difference is that interfaces are merely "contracts", they only list the methods that should be available for that type, without actually implementing them. If a variable has an interface type (e.g. List
is such an interface), then it should be assigned to an object which is an instance of a class that actually defines/implements those methods. E.g.: List myList = new ArrayList();
, or List myList = new LinkedList();
, or List myList = new Vector();
. The type of myList
in all the above cases is List
, and can be used as a List
everywhere, but its actual value is a different type of object, a different (sub)type of List
, which could have additional methods on top of those required by the List
"contract".
The flexibility provided by interfaces comes in handy e.g. when passing arguments to functions - if we give a parameter an interface type instead of a specific class, then the function can accept many different implementations/subtypes. A silly function for illustration (note: the compiler will give raw type warnings if you run this, but don't worry about them - I did not want to complicate the thing with generics yet):
import java.util.List;
import java.util.ArrayList;
import java.util.LinkedList;
class InterfaceExample {
// this accepts ArrayLists only
static void printFirstElement(ArrayList myList) {
System.out.println(myList.get(0));
}
// this accepts any List arguments
static void printFirstElementPoly(List myList) {
System.out.println(myList.get(0));
}
public static void main(String[] args) {
ArrayList al = new ArrayList();
al.add("hello");
LinkedList ll = new LinkedList();
ll.add("hello");
printFirstElement(al); // works
//printFirstElement(ll); --> compile time error - we're passing in a LinkedList, but this only accepts ArrayLists
// however
printFirstElementPoly(al); // works
printFirstElementPoly(ll); // also works!
}
}
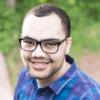
Philip Gales
15,193 PointsHe may have briefly mentioned it. But you would chain together a bunch of methods like this:
myNameVariable.toLowerCase().removeVowels()
When you use a method, the object that goes before the dot has to be a certain type. I can't pass in a variable of type 'int' and do that, I had to pass in a variable of type 'String'.
Continue learning java and practicing and it will make more sense.

Dee Palmer
688 PointsWe may have done method chaining in Java Basics, but he did not call it method chaining. This refresher is essentially all new material.
Joshua Compton
4,450 PointsJoshua Compton
4,450 PointsWow. Super helpful explanation. Thank you very much.
Yusuf Mohamed
2,631 PointsYusuf Mohamed
2,631 PointsLove you answer but I have a question. What is an example that you can put in where you used three dots in stead?
gyorgyandorka
13,811 Pointsgyorgyandorka
13,811 PointsYusuf Mohamed I just meant assignment to some variable, I edited my answer, sorry.
Yusuf Mohamed
2,631 PointsYusuf Mohamed
2,631 PointsGyorgy Andorka Thank you for the clarification.