Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial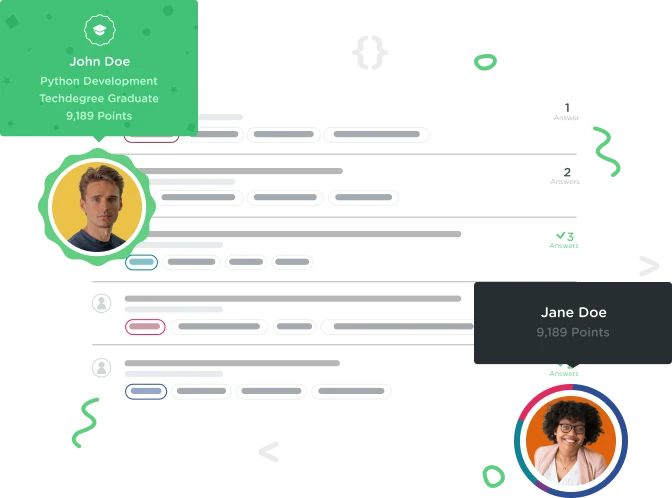

Dave Masom
9,584 PointsRemove all instances of a value from a list
Is there a way to remove all instances of a value in a list? For example, if I have: my_list = [1, 2, 3, 3, 3]
my_list.remove(3) results in my_list = [1, 2, 3, 3].
Can I call a method to remove all of the 3s in my_list?
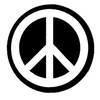
john larson
16,594 PointsSeth, it looks like yours is creating a new list out of only the desired characters? Kinda like the opposite of what I did?
2 Answers
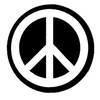
john larson
16,594 PointsThis seemed to work. Though I'm sure there are more graceful ways to do it. I'll be curious what someone else will suggest.
for num in my_list:
my_list.remove(3)

Seth Kroger
56,413 Pointsmy_list = [x for x in my_list if x != 3]
works too

Dave Masom
9,584 PointsThanks John, it worked for me too. And I understood your solution as well - even better!
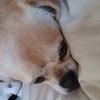
John Schaub
5,167 PointsI tried both methods and Seth's ended with the results intended and John's left me with a syntax error! not sure what I did wrong?
my_list = [54321, 3, 3, 3]
my_list
[54321, 3, 3, 3]
for num in my_list: my_list.remove(3)
... my_list
File "<stdin>", line 2
my_list
^
SyntaxError: invalid syntax
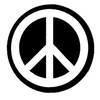
john larson
16,594 PointsI didn't get a syntax error, but it left one 3 when running the code on you're list. Seths code is cooler anyway :D Ok, I ran my code in the python shell and got that syntax error, but it did remove all the 3's. So I would have to add some more code to clean it up. Seths is still better.

ryan smith
687 PointsWhat does x for x in my_list mean? I didn't understand your solution.

Seth Kroger
56,413 PointsIt's called a list comprehension. Comprehensions are kinda unique to Python (it is the only major language to have them). It's a bit like a for loop but it basically means "make a new list but running through the items of the old list and checking if they meet a condition". You can check out this workshop for more: https://teamtreehouse.com/library/python-comprehensions
john larson
16,594 Pointsjohn larson
16,594 PointsHi Dave, glad to help. It took me a few minutes to figure it out and when I got it I was pleasantly surprised.