Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial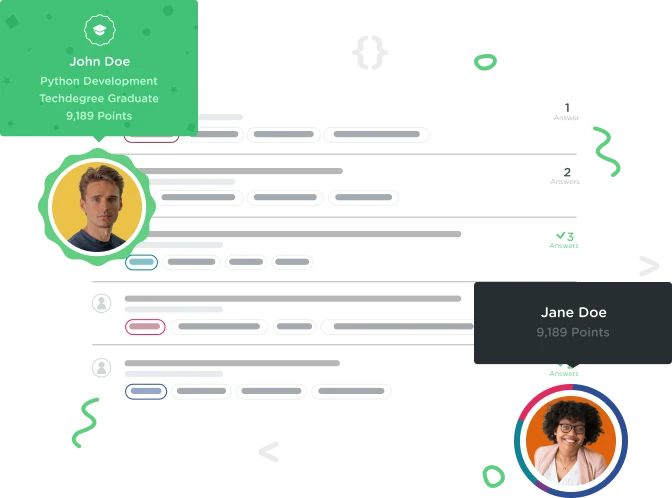
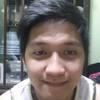
Errol Gonzales
20,948 Pointsremove char or String from a String?
I'm just wondering, if you can concatenate a String to a String or char. how can you remove a char or String from a String???
1 Answer
Christopher Augg
21,223 PointsErrol,
Actually, you cannot change a String at all. When you concatenate a String, you are actually creating a new String object. There are a lot of methods within the String class that can help you achieve what appears as adding or removing to a String, but Strings are immutable (cannot be changed). You can see this in the String class documentation.
Here is an example of a few of the methods. Please try using others within the documentation provided.
public class MAIN {
public static void main(String[] args) {
String name = "This is a String";
name.concat(" concatenated with this String.");
System.out.println("Not changed: " + name);
name = name.concat(" concatenated with this String.");;
System.out.println("Actually we create a new String with concatenation: " + name);
name = name.substring(0, 16);
System.out.println("Choose start and end index of String: " + name);
name = name.substring(10);
System.out.println("Start at 0 and go to 10: " + name);
char letter = name.charAt(0);
System.out.println("Getting a character does not change String: " + name);
System.out.println("Character: " + letter);
}
}
I hope this helps. Please let me know if I can be of further assistance.
Regards,
Chris
Errol Gonzales
20,948 PointsErrol Gonzales
20,948 PointsChris,
Thank you, it helps a lot!