Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial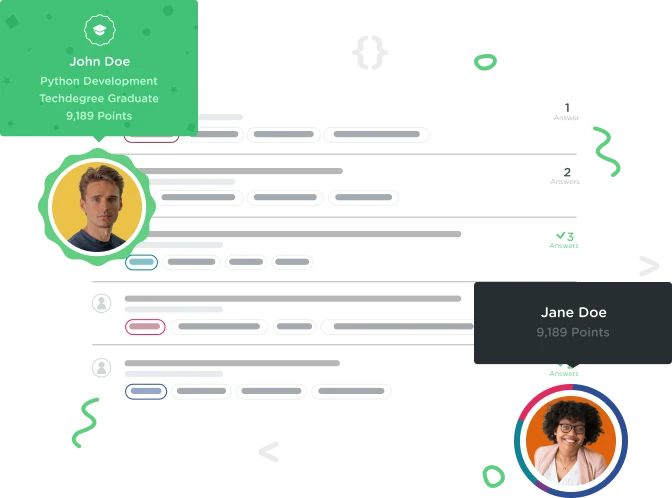

bhuvaneswari seshadri
134 Pointsremove function is not removing some characters
def disemvowel(word): word = list(word) for letter in word: if letter =="u" or letter == "U": word.remove(letter) elif letter == "a" or letter == "A": word.remove(letter) elif letter == "e" or letter == "E": word.remove(letter) elif letter == "o" or letter == "O": word.remove(letter) elif letter == "i" or letter == "I": word.remove(letter)
return word
def disemvowel(word):
word = list(word)
for letter in word:
if letter =="u" or letter == "U":
word.remove(letter)
elif letter == "a" or letter == "A":
word.remove(letter)
elif letter == "e" or letter == "E":
word.remove(letter)
elif letter == "o" or letter == "O":
word.remove(letter)
elif letter == "i" or letter == "I":
word.remove(letter)
return word
1 Answer

Wade Williams
24,476 PointsYou have a bug in your code and this is a great way to learn to about this particular bug as it's not always obvious. The problem is you're changing the "word" variable as you're looping over it. So effectively, every time you remove a vowel, all the letters shift down 1 index and your loop skips the letter next to the vowel, which is why you're missing letters.
Here is a simple solution using your code base. We need to assign another variable to list(word) which will act as our control variable, then we can remove items from "word" without affecting our loop variable. Also, after the loop you need to join your loop so you're returning a string and not a list.
def disemvowel(word):
word = list(word)
letter_list = list(word)
for letter in letter_list:
if letter =="u" or letter == "U":
word.remove(letter)
elif letter == "a" or letter == "A":
word.remove(letter)
elif letter == "e" or letter == "E":
word.remove(letter)
elif letter == "o" or letter == "O":
word.remove(letter)
elif letter == "i" or letter == "I":
word.remove(letter)
return "".join(word)