Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial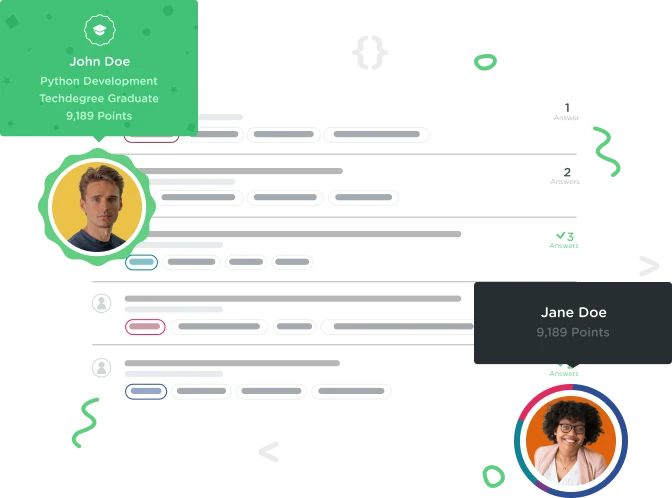

Script Code
6,631 Pointsremove method removes 1 instead of True from list
If you have a list with a Boolean value True at index 0 and int 1 at index 1. How do you remove True using the remove method?
my_list = [1, True] my_list.remove(True) This returns [True]
I am aware that 1 == True returns True and that; 1 is True returns False False
Because 1 and True are not the same objects id(1) returns 1697929488 whilst, id(True) returns 1697748080
However I am not able to think of a way to perform the above. HELP!! :)
3 Answers
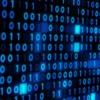
Alexander Davison
65,469 PointsIf you want to delete an item in a list, there's two ways.
One is by index, the other way is to do it by element. Here's some examples:
# Here's a list of the alphabet
my_list = list('abcdefghijklmnopqrstuvwxyz')
# This is the index way of deleting items
del my_list[0] # This will delete "a" because "a" is at index 0.
# This is the element way of deleting items
my_list.remove('z') # This will remove "z" from the list.
so, to solve your problem, you could do this:
your_list = [True, 1]
# This will delete True
your_list.remove(True)
Hope it helps! :) ~Alex

Script Code
6,631 Points- your_list = [True, 1]
- your_list.remove(True) # This returns [1]
However (1 and True in different order):
- my_list = [1, True]
- my_list.remove(True) # This returns [True]
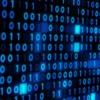
Alexander Davison
65,469 PointsHmm... That's strange. Try deleting by indexes like this then:
your_list = [True, 1]
del your_list[0]
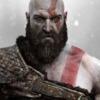
boi
14,242 PointsFor Future audience.
The reason you are getting True
in return is because True == 1
There are two scenarios here, the first case scenario
your_list = [True, 1]
your_list.remove(True) #Returns [1]
The second case scenario
my_list = [1, True]
my_list.remove(True) # Returns [True]
1
is considered True
and 0
is considered False
, Also note that the remove
function, if used on a list having duplicate objects, will remove the first duplicate or will remove them in order, The above case scenarios proofs this behavior.
Try these exercises
my_list = [1, True]
print(sum(my_list)) #The function "sum" returns the summation of the objects in the list
my_list = [False,True]
print(sum(my_list)) #The function "sum" returns the summation of the objects in the list
Script Code
6,631 PointsScript Code
6,631 PointsHi Alex,
But what if the list order is [1, True]? Does this mean I have to reorder the list before I can use the remove method?
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsBig no no! This code will still work because I'm telling Python to search for True in your list and if there is one, Python will delete it.