Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial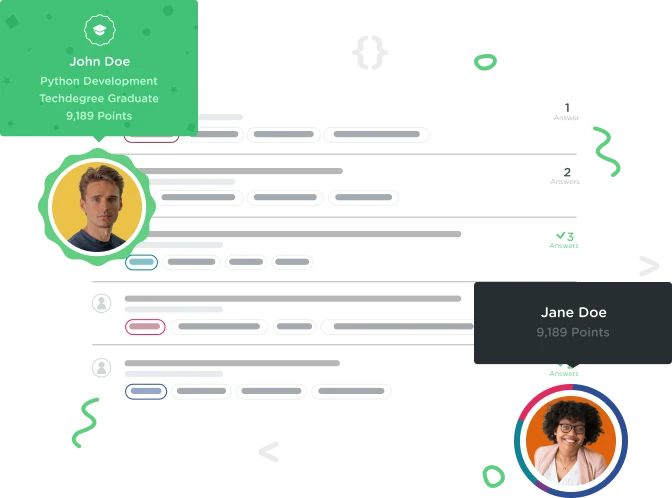

Jason Ngo
6,947 PointsremoveInvitee not removing element
const selenium = require('selenium-webdriver');
const By = selenium.By;
const driver = new selenium.Builder().forBrowser("chrome").build();
driver.get(process.env.URL);
const invitees = [
'Gonzalo Torres del Fierro',
'Shadd Anderson',
'George Aparece',
'Shadab Khan',
'Joseph Michael Casey',
'Jennifer Nordell',
'Faisal Albinali',
'Taron Foxworth',
'David Riesz',
'Maicej Torbus',
'Martin Luckett',
'Joel Bardsley',
'Reuben Varzea',
'Ken Alger',
'Amrit Pandey',
'Rafal Rudzinski',
'Brian Lynch',
'Lupe Camacho',
'Luke Fiji',
'Sean Christensen',
'Philip Graf',
'Mike Norman',
'Michael Hulet',
'Brent Suggs'
];
const locators = {
inviteeForm : By.id("registrar"),
inviteeFieldName: By.css("#registrar input[name='name']"),
toggleNonresponders: By.css(".main > div input"),
removeButtonForInvitee: invitee => By.xpath(`//span[text()="${invitee}"]/../button[last()]`)
}
function addInvitee(name){
driver.findElement(locators.inviteeFieldName).sendKeys(name);
driver.findElement(locators.inviteeForm).submit();
}
function removeInvitee(invitee){
driver.findElement(locators.removeButtonForInvitee(invitee)).click();
}
function toggleNonrespondersVisibility(){
driver.findElement(locators.toggleNonresponders).click();
}
invitees.forEach(addInvitee);
removeInvitee("Shadd Anderson");
1 Answer
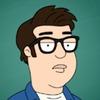
Unsubscribed User
15,444 PointsUnfortunately, I can't answer why it isn't work, but I'm having the same issue. So thought I'd share my insight.
It looks as though something has changed, the script that was working well for Craig is now seems to be completing 'too quickly'.
When I enter the script line by line into the REPL - everything works fine.
Frustrating that I have to manually run an automation script line by line in order for it to work!
If anyone could provide some insight on how we can rectify this, that would be much appreciated.
Jason Ngo
6,947 PointsJason Ngo
6,947 PointsI was able to figure out what the issue is. The version Craig is using is Selenium 3.6 and the version I have installed is 4.0. If you want to have the code work in version 4.0 you will need to do an implicit or explicit wait otherwise Craig's code will not work correctly.
Use this command in your terminal to install version 3.6 and all of the tutorial's code should work correctly. npm install selenium-webdriver@3