Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial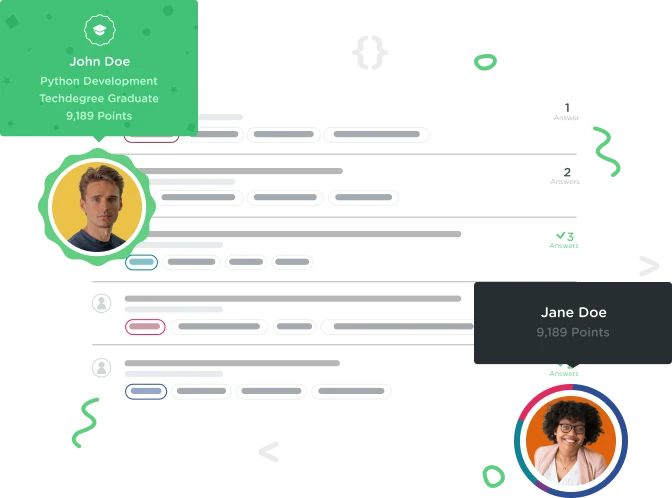

Idris Abdulwahab
Courses Plus Student 2,961 Pointsremoving items from a list
Quiz from code challenge: OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end. I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you! Oh, be sure to look for both uppercase and lowercase vowels!
def disemvowel(word):
base = list(word)
vowels = "aeiou"
small = vowels.lower
big = vowels.upper
for char in base:
for vowel in vowels:
if char == small or big:
base.remove(char)
word = "".join(base)
return word
3 Answers
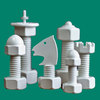
Steven Parker
231,269 PointsPerhaps these hints will help:
- when calling a method (like "lower()"), the method name should be followed by parentheses
- when combining tests with "and"/"or", both sides must be complete comparison expressions
- removing items from an iterable inside the loop that uses it can cause other items to be skipped over

Idris Abdulwahab
Courses Plus Student 2,961 PointsHi Steven, Thank you for your help but I couldn't figure it out. I used the hints you provided but still not getting through. Please spill it out. Thank you.
def disemvowel(word):
base = list(word)
vowels = "aeiou"
small = vowels.lower()
big = vowels.upper()
for char in base:
if char == small or char == big:
base.remove(char)
word = "".join(base)
return word
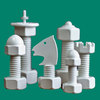
Steven Parker
231,269 PointsWhen posting code, use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.

Idris Abdulwahab
Courses Plus Student 2,961 PointsThank you so much for your help and hints. I learnt from it and I do appreciate it.
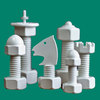
Steven Parker
231,269 PointsIdris Abdulwahab — Normally you'd select "best answer" as the one that helped the most to resolved the issue. Choosing one that actually contains only a comment may be confusing to other readers.
And did that last suggestion help you to resolve the issue?
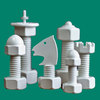
Steven Parker
231,269 PointsYou're still removing from "base" while using it as the loop iterator. To avoid skipping over items, try using a copy as the iterator. A slice might be handy for that ("base[:]
").