Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial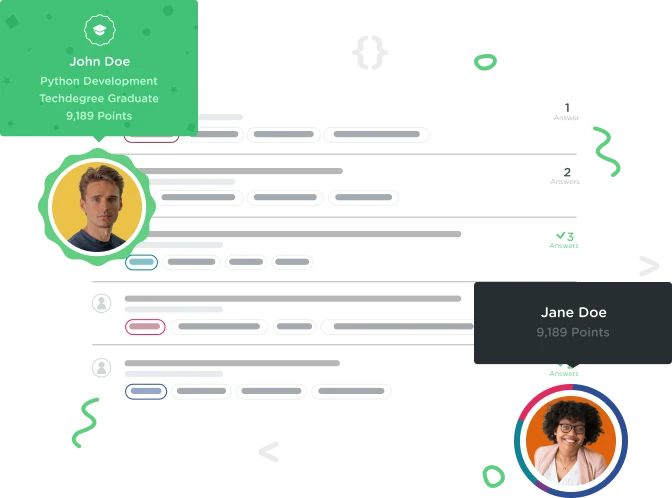

Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsRemoving Items From Array
I wanted to delete a specific element within the array not first or last element. So I write the solution as-
var arr = [2, 3, 5, 6];
var itemIndex = arr.indexOf(3);
arr.splice( itemIndex , 1);
But when I searched to find , is it the correct one. I found this solution-
var array = [2, 5, 9];
var index = array.indexOf(5);
if (index > -1) {
array.splice(index, 1);
}
So my question is "why condition statement is used here?". What are the conditions where "indexOf() function can return negative index?" . Please explain . Thankyou.
1 Answer
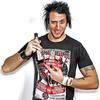
Andreas Nyström
8,887 PointsHi.
The indexOf-function returns -1 if it doesn't find an index. So if you try to look for 33 in your code your index would be -1. The conditional is there to prevent it from splicing if you didnt find an index. If you don't have it, you will splice the LAST item in your array.
See this example:
let myArray = [3, 55, 4]
// index is now set to -1 because it didn't find the number in the array.
let index = myArray.indexOf(344);
// This will now remove 4 in the array as it is the last item of the array.
// Because it didnt find an index.
// This is not what you wanted.
myArray.splice(index, 1);
// Instead we're using a condintional statement
// Because we only want the splice to happen if an index was found
if (index > -1) {
myArray.splice(index, 1);
}
Aakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsAakash Srivastav
Full Stack JavaScript Techdegree Student 11,638 PointsOhkay Andreas . Thanks a lot man.... :)