Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial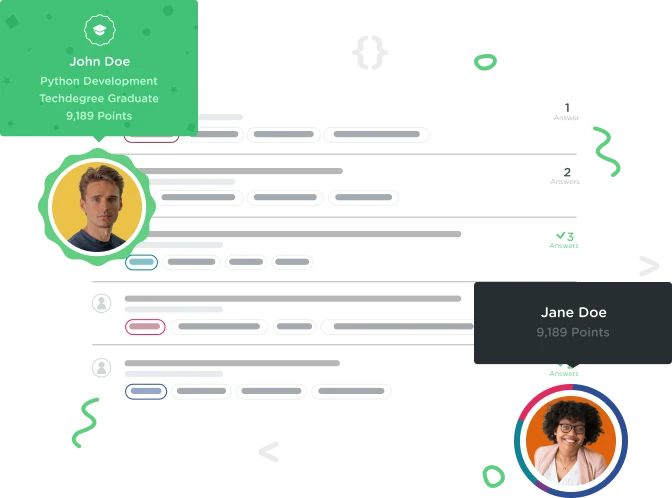

Heather Stone
5,784 Pointsremoving items from JavaScript array and getting "...is not a function" error
I'm trying to iterate over an array of phone numbers and remove the dashes. When I run this code, I get an error message saying "fullNumberList.replace is not a function". Why? Thanks in advance for your help.
var fullNumberList = ["111-222-3333", "444-555-6666"];
for(var i = 0; i < fullNumberList.length; i++) {
var dashless = fullNumberList.prototype.replace("-", "");
console.log(dashless);
}
1 Answer

Arturo Alviar
15,739 PointsOkay so I am not sure if you copied all you code but your for loop is a little wired.
Also you are using the replace function incorrectly.
What fullNumberList.prototype.replace() means is that you gave the variable a function called replace. In addition, replace() works with strings only so doing the below would raise an error.
fullNumberList.replace("-", " "); //Uncaught TypeError: fullNumberList.replace is not a function(…)
Here is something you can use:
for(var i = 0; i < fullNumberList.length; i++){
//iterate through the fullNumberList array and split each element into arrays if string has a -
//the split function removes the - from the string since the string is now broken into smaller strings
//after the - is gone, join the string and add a space in between
console.log(fullNumberList[i].split("-").join(" "));
}
This code just prints the new strings but I am sure you can use this to get to your goal.
Hope this helps!