Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial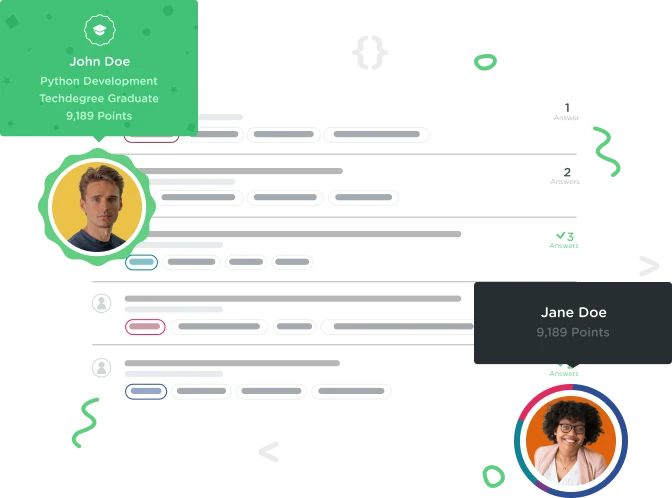

Brian York
6,213 PointsRemoving letter from word
Hi,
Here is the prompt from my code challenge: "OK, I need you to finish writing a function for me. The function disemvowel takes a single word as a parameter and then returns that word at the end. I need you to make it so, inside of the function, all of the vowels ("a", "e", "i", "o", and "u") are removed from the word. Solve this however you want, it's totally up to you! Oh, be sure to look for both uppercase and lowercase vowels!"
My code is syntactically fine (I'm not getting errors), but I am not getting the results I want, and am not sure why. I've seen other people post different ways to complete this challenge, but none of them use the .remove method, which was featured in the video before the challenge, so I am attempting to do that. Should I be using something else?
Thanks, Brian
def disemvowel(word):
a = True
letter = ['a', 'e', 'i', 'o', 'u',]
lower_word = word.lower()
my_list = list(lower_word)
for let in letter:
while a == True:
try:
my_list.remove(let)
except ValueError:
a = False
word = ''.join(my_list)
return word
7 Answers
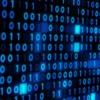
Alexander Davison
65,469 PointsI think you are over-thinking this challenge.
You should try this:
def disemvowel(s):
result = ''
for letter in s:
if letter.lower() not in 'aeiou':
result += letter
return result
How this works:
- We make our function and set a variable called
result
to an empty string. This is useful for later where we add letters onto the string (or "growing" the string) - We loop through all the letters in the string passed in to the function.
- If the current letter lowercased is not in 'aeiou', we will "grow" the string.
- After the loop is over, we return the completed string.
I hope this helps. ~Alex
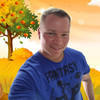
benjamingarrison
1,649 PointsDisclaimer
The following code will solve the challenge for you. It took me a few hours to figure it out, which was a lot of fun learning a new method called replace(). I would suggest looking into the the function replace() before simply copy and paste. Try solving it with this tip.
I have found a more efficient way, using "Regular Expressions". I will re-visit this once I understand that concept more.
Solution:
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u']
for letter in word:
if letter.lower() in vowels:
word = word.replace(letter, "")
return word
print(disemvowel("This is a test"))# Don't include this in challenge
Output:
Ths s tst
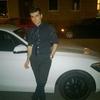
Cristiano Monteiro
5,942 Pointshi i was wondering why when i write this like in your code: word = word.replace(letter, ""), it passes but if i write only word.replace(letter, "") it doesnt. Everything else is the same

frank sebring
1,037 PointsThe following code will work to solve the problem but I wanted to know if someone knew a way to condense the while statements in step 3 to one statement instead of ten. Thanks
def disemvowel_my_method(word): #Step 2: word_list = list(word) print(word_list)
#Step 3:
while "a" in word_list:
word_list.remove("a")
while "A" in word_list:
word_list.remove("A")
while "e" in word_list:
word_list.remove("e")
while "E" in word_list:
word_list.remove("E")
while "i" in word_list:
word_list.remove("i")
while "I" in word_list:
word_list.remove("I")
while "o" in word_list:
word_list.remove("o")
while "O" in word_list:
word_list.remove("O")
while "u" in word_list:
word_list.remove("u")
while "U" in word_list:
word_list.remove("U")
print(word_list)
#Step 4:
new_word = "".join(word_list)
print(new_word)
Method given on internet
def disemvowel(word): word_as_list = list(word) vowels=["a","e","i","o","u","A","E","I","O","U"] for char in vowels: while True: try: word_as_list.remove(char) except ValueError: break return ''.join(word_as_list)
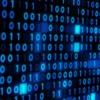
Alexander Davison
65,469 PointsMaybe you can try this:
def disemvowel(word):
word_as_list = list(word)
vowels = list('aeiouAEIOU')
for vowel in vowels:
while vowel in word_as_list:
word_as_list.remove(vowel)
return ''.join(word_as_list)
I hope this helps

Brian York
6,213 PointsYeah, I guess I was overthinking it. Thanks, this solution makes sense.
Chris Grazioli
31,225 Pointsdef disemvowel(word):
vowels=["a","e","i","o","u","A","E","I","O","U"]
for char in vowels:
while True:
try:
word.remove(char)
except ValueError:
return False
return word
Any thoughts on how to make this work? I think its close...
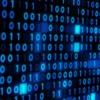
Alexander Davison
65,469 PointsYou are indeed close. First, though, why are you returning False
in the except ValueError
block? I don't think the challenge asked you to return anything but a string.
Second, remove
isn't a method on strings. So what I did is that I converted the variable to a list and assigned that to a new variable, then called remove
on that list instead of the string.
Finally, since I don't want to return a list, I joined it together with no symbols with ''.join(word_as_list)
. Think of ''.join(word_as_list)
as word_as_list.join('')
. It makes a little more sense that way.
Fixed version of your code:
def disemvowel(word):
word_as_list = list(word)
vowels=["a","e","i","o","u","A","E","I","O","U"]
for char in vowels:
while True:
try:
word_as_list.remove(char)
except ValueError:
break
return ''.join(word_as_list)

Christopher Kehl
18,180 PointsTruthfully, I'm not liking this exercise. It leaves out too may details.

wads wdsf
Python Web Development Techdegree Student 1,678 PointsOne line solution: def disemvowel(word): word=''.join([i for i in word if i.lower() not in ['a','e','i','o','u']]) return word
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsYour welcome :)
Cristiano Monteiro
5,942 PointsCristiano Monteiro
5,942 Pointsi am not understand your solution at all would you mind going through for "Dummies" ?
Pavlo Morgun
18,688 PointsPavlo Morgun
18,688 PointsThis makes a lot of sense.
I was removing a letter from the word and then looping over it. Caused me an issue because the length of the word was decreasing so I couldn't iterate over it fully.
christopher abjornson
5,572 Pointschristopher abjornson
5,572 PointsThat helped a lot and i love that way you broke it down,