Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial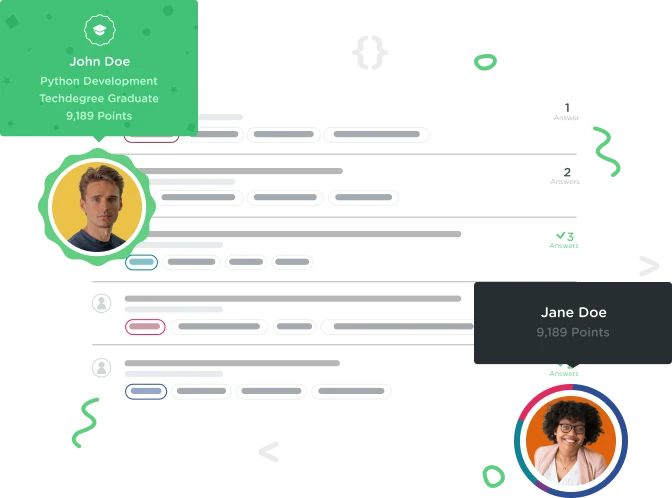

Trevor McGill
1,409 PointsRemoving letters from word function
I’m having issues with the challenge that asks us to remove vowels from a user’s entry. I’ve created a loop to go through the user’s entry and find the vowels to remove it, but I can’t get the function to take out uppercase vowels even when I tried to use letter.upper().
Suggestions?
def disemvowel(word):
for letter in word:
if ('a' or 'e' or 'i' or 'o' or 'u') in letter:
del letter
return word
2 Answers
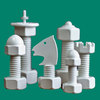
Steven Parker
231,269 PointsLogic operators can be used to combine complete expressions, but not parts of expressions. For example:
if letter == ("a" or "b"): # this will not match any letter
if letter == "a" or letter == "b": # but this will
Also, deleting a loop variable ("del letter
") does not have any effect on the loop iterable
And one final hint: "return word
" just returns the original word unmodified.

Trevor McGill
1,409 PointsOkay, so I tried returning a new string per your recommendations, and I am still not seeing changes when the value is returned. Here’s my code:
def disemvowel(word):
try:
new_word = word.replace('a''e''i''o''u''A''E''I''O''U','')
return new_word
except ValueError:
return new_word
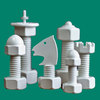
Steven Parker
231,269 PointsYou won't need "try/except" for this. And the "replace" function only works on things that match exactly, so if your string has all the vowels it will only remove them if they are also all together in the word.
You may want to use a loop to remove one at a time.
Trevor McGill
1,409 PointsTrevor McGill
1,409 PointsThank you for your hint. Why would the del keyword not work in this situation?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsAs I said, deleting the loop variable doesn't have any impact on the original word, but even more important is that Python strings are immutable (they cannot be modified).
You can, however, construct a new string that is a modified copy of the original. The string function "replace" might come in handy.