Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial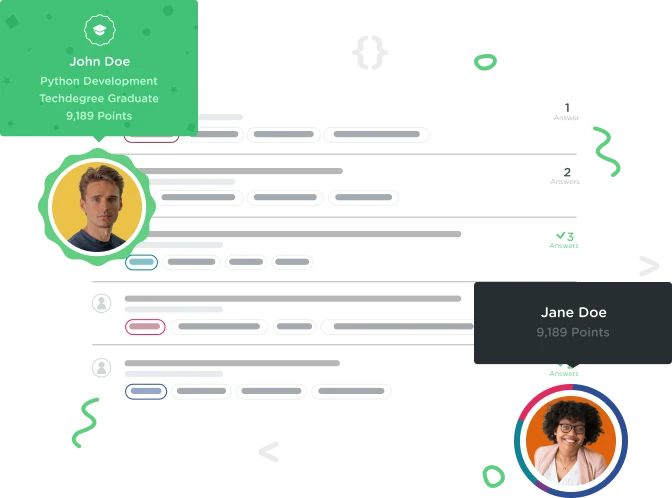
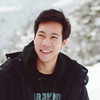
Howie Yeo
Courses Plus Student 3,639 PointsRemoving more than 1 element using pop and shift method?
Hi, I am wondering if there is a way to remove more than 1 element from the array using pop and shift method?
3 Answers

ericb2
11,429 PointsYou could mimic the behavior of .pop()
or .shift()
for multiple elements using .splice()
(not to be confused with .slice()
):
arrayName.splice(startIndex, deleteHowMany)
To return elements deleted from the beginning, use a start index of 0:
var arr = ['a', 'b', 'c', 'd'];
var first2 = arr.splice(0, 2); // first2 is [ "a", "b" ]
// arr is now [ "c", "d" ]
To return elements deleted from the end, use a negative index (-1 for last, -2 for next-to-last, etc):
Array [ "a", "b", "c", "d" ]
var last2 = arr.splice(-2, 2); // last2 is [ "c", "d" ]
// arr is now [ "a", "b" ]
here is a link to the MDN doc page for splice.
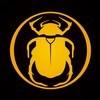
rydavim
18,814 PointsYou could use a loop to remove multiple items from an array using pop and shift, but each call may only remove a single element.
If you'd like to remove multiple elements perhaps splice might work better for you?

Anuj Sachdeva
13,034 PointsYou can create a function for this purpose. Like I did here :
var numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9];
function removeItems(arr, item) {
for ( var i = 0; i < item; i++ ) {
arr.pop();
}
}
removeItems(numbers, 2);
Howie Yeo
Courses Plus Student 3,639 PointsHowie Yeo
Courses Plus Student 3,639 PointsThanks ericb2, your explanation is really clear!