Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial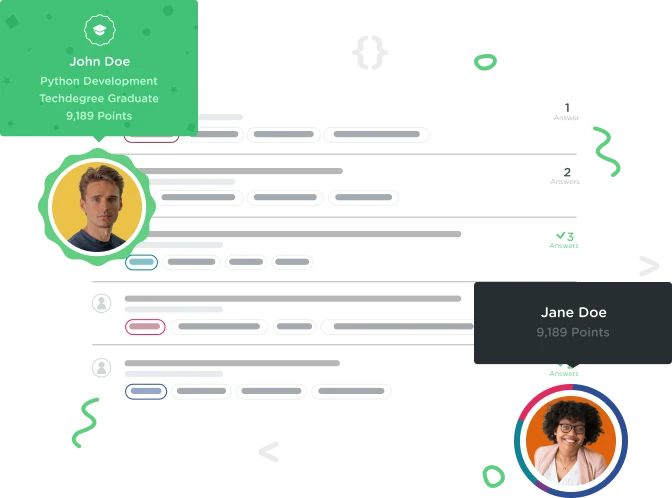

Neil Bircumshaw
Full Stack JavaScript Techdegree Student 14,597 PointsRemoving multiple select elements
What I'm trying to do is when one select option is selected with a particular piece of text, then another select element appear with different options according to what was picked in the first select element.
The problem I'm having is when I'm removing the select element so that it doesn't show up next to another select element it will not work because it's either already been removed from the DOM and is not a child element anymore or has not yet been appended.
Here is my code for this part of the problem:
const color_div = document.getElementById("colors-js-puns")
const color = document.getElementById("color")
const design = document.getElementById("design")
const select_1 = document.createElement("select")
const please_select_option = document.createElement("option")
const select_2 = document.createElement("select")
const Cornflower_Blue = document.createElement("option")
const Darkslategrey = document.createElement("option")
const Gold = document.createElement("option")
const select_3 = document.createElement("select")
const Tomato = document.createElement("option")
const Steelblue = document.createElement("option")
const Dimgrey = document.createElement("option")
please_select_option.value = "please_select";
please_select_option.textContent = " << Please select a design!";
Cornflower_Blue.value = "Cornflower Blue";
Cornflower_Blue.textContent = "Cornflower Blue";
Darkslategrey.value = "Darkslategrey";
Darkslategrey.textContent = "Darkslategrey";
Gold.value = "Gold";
Gold.textContent = "Gold";
Tomato.value = "Tomato";
Tomato.textContent = "Tomato";
Steelblue.value = "Steelblue";
Steelblue.textContent = "Steelblue";
Dimgrey.value = "Dimgrey";
Dimgrey.textContent = "Dimgrey";
color_div.appendChild(select_1);
select_1.appendChild(please_select_option);
color_div.removeChild(color);
design.addEventListener("change", () => {
if(design.value === "js puns"){
color_div.appendChild(select_2);
select_2.appendChild(Cornflower_Blue);
select_2.appendChild(Darkslategrey);
select_2.appendChild(Gold);
color_div.removeChild(select_3);
color_div.removeChild(select_1);
}
else if (design.value === "heart js"){
color_div.appendChild(select_3);
select_3.appendChild(Tomato);
select_3.appendChild(Steelblue);
select_3.appendChild(Dimgrey);
color_div.removeChild(select_2);
color_div.removeChild(select_1);
}
else {color_div.appendChild(select_1);
color_div.removeChild(select_3);
color_div.removeChild(select_2);}
});
the problem lies here:
design.addEventListener("change", () => {
if(design.value === "js puns"){
color_div.appendChild(select_2);
select_2.appendChild(Cornflower_Blue);
select_2.appendChild(Darkslategrey);
select_2.appendChild(Gold);
color_div.removeChild(select_3);
color_div.removeChild(select_1);
}
because select_3 does not exist yet - it hasn't yet been appended to the DOM, an error message appears, however I need it there to remove it later when it does get appended, or else it will just stay there as an extra input that's not needed on the page. Any tips would be great!
2 Answers
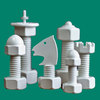
Steven Parker
231,236 PointsHow about leaving everything on the page and alter visibility?
Instead of adding and removing the elements, you could have them all on the page at all times and just change their display properties to either none (invisible) or block (visible). Then you'd never have a case where you try to reference one that doesn't exist.

Neil Bircumshaw
Full Stack JavaScript Techdegree Student 14,597 PointsYeah, I've just done it now...I can't believe it was that simple. For some reason in my head I thought that changing an element's display to "none" still meant it was on the page and that elements would be pushed next to it as a sibling - almost that it gives the impression it's gone, but it's still actually there, whereas removeChild fully removes it from the DOM. I'm guessing setting it's display to "none" is as good as removing it.
Thanks a lot Steven, you're a massive help.
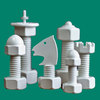
Steven Parker
231,236 PointsYou might be thinking of "visibility:hidden
" — that hides the element but it still takes up the same space as if it were shown. But with "display:none
" it also does not take up any space. Happy to help!