Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial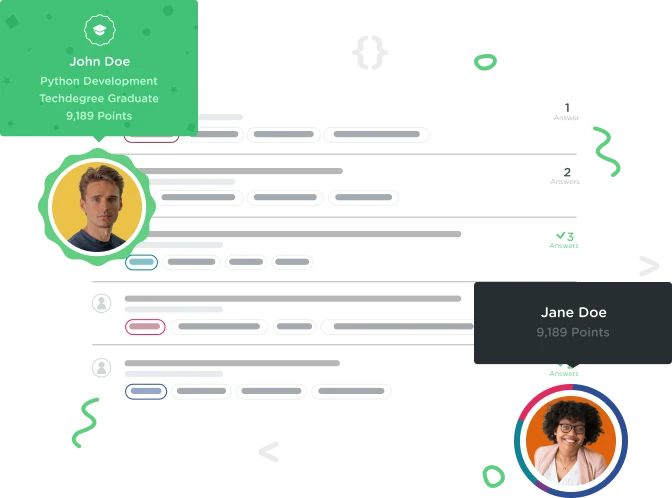

Tony Nguyen
24,934 PointsRemoving negative numbers from a given array.
Hello Fellow Students!
I am trying to solve an algorithm challenge that I was provided. I understand that there are helper JavaScript Methods I can use however I am very limited.
The only methods I am allowed to use or .push, .pop, and .length and I am not allowed to store a temporary array in a variable.
Here is what I have so far but it doesn't solve the problem logically.
var x = [-3, 5, 1, 3, 2, 10];
function removeNegative(a) {
var first = 0;
var last = a.length - 1;
while (first < last) {
if (a[first] < 0) {
var temp = a[first];
a[first] = a[a.length - 1];
a[a.length - 1] = temp;
a.pop();
}
first++;
last--;
}
return a;
}
console.log(removeNegative(x)); //Returns [10, 5, 1, 3, 2]
The problem with this is that it reverses my array when I don't want it to.
It removes the first negative number that I wanted to remove initially, however if I were to add a negative number at the end, it would not be removed but instead be placed in the beginning of the array.
Thank you in advance!

Tony Nguyen
24,934 PointsI'm not allowed to use something like this:
var x = [];
Other temporary variable storage is okay!
1 Answer
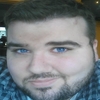
Marcus Parsons
15,719 PointsOkay cool, well I came up with something that only uses length and will only grab out negative numbers from the array, regardless of position. Although since you can't use a temporary container, x permanently changes, of course. This is unavoidable without a temporary container.
var x = [-3, 5, 1, 3, 2, 10];
function removeNegative (arr) {
for (var i = 0, j = 0, l = arr.length; i < l; i++) {
if (arr[i] >= 0) {
arr[j++] = arr[i];
}
}
arr.length = j;
return arr;
}
console.log(removeNegative(x));

Tony Nguyen
24,934 PointsAwesome thanks! You are the master! I've been trying to figure this out for like an hour then I had to seek teamtreehouse for help.
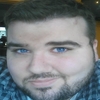
Marcus Parsons
15,719 PointsYou are very welcome! :D Do you have any questions about how it works?

Tony Nguyen
24,934 PointsActually I have a general question. So i'm really trying to get a hang of solving algorithms. But how do you really get good at it? Did you do this all in your head or did you have to use a piece of paper and wrote pseudo code to help you think of it?
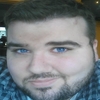
Marcus Parsons
15,719 PointsPersonally, I don't really write anything on paper anymore because I hate wasting paper haha. But, I do tend to type out pseudocode to solve things. I'm as specific as I can be when writing out the problem and also when outlining possible solutions. And then as I'm typing out the solution, I do lots and lots of experimentation.
This is actually the first time I've solved an algorithm challenge like this. I don't count the previous one because you came up with the base code; I just helped modify it to work :P

Tony Nguyen
24,934 PointsActually, as I'm trying to read through the code, I am having a hard time trying to figure out how it works. May I ask you how it is removing the negative number from the array?
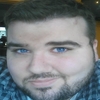
Marcus Parsons
15,719 PointsWell, we're starting with two variables that are controlling indices in the array. You have i which is the regular iterator controller and j which is the sub iterator controller. i goes over each index in the array, of course. At each index, the if statement checks to see if the value stored at that index is greater than or equal to 0. If it is, then it takes and sets the value at index j to the value stored at i and then increases j by 1.
So, if the if-statement does not go off, j will not increase, and so when another positive number is found, j will be at the last location before it found a positive number and it will overwrite that location with the positive number found in the next location.
The array gets overwritten many times until you end up with an array with extra copies of values at the end of the array. By resetting the array length to j's value (which will be behind i if any negative numbers are found), we get rid of any extra values on the end because the new array is going to have less indices than the old one since we got rid of any negative numbers at those indices.
For example, in your array there are 6 indices, but since we wanted to get rid of the negative numbers, the new version of the array should only have 5 indices (the amount of positive numbers in the array and the value of j).
Run this in your console (added some console.log statements):
var x = [-3, 5, 1, 3, 2, 10];
function removeNegative (arr) {
for (var i = 0, j = 0, l = arr.length; i < l; i++) {
if (arr[i] >= 0) {
console.log('j before assignment: '+j);
arr[j++] = arr[i];
console.log('j after assignment: '+j);
console.log('arr is currently: '+arr);
}
}
arr.length = j;
return arr;
}
console.log(removeNegative(x));

Tony Nguyen
24,934 PointsAwesome explanation. Thank you so much!
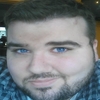
Marcus Parsons
15,719 PointsAnytime, Tony! Good luck, man!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsHey again Tony,
I need to clarify that last bit of the challenge again "I am not allowed to store a temporary array in a variable." You were storing information from the array in a variable in the last challenge and you had this text as well. Do you mean to say instead that you can't store the array as a temporary array instead of saying you can't store the array into a variable?