Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial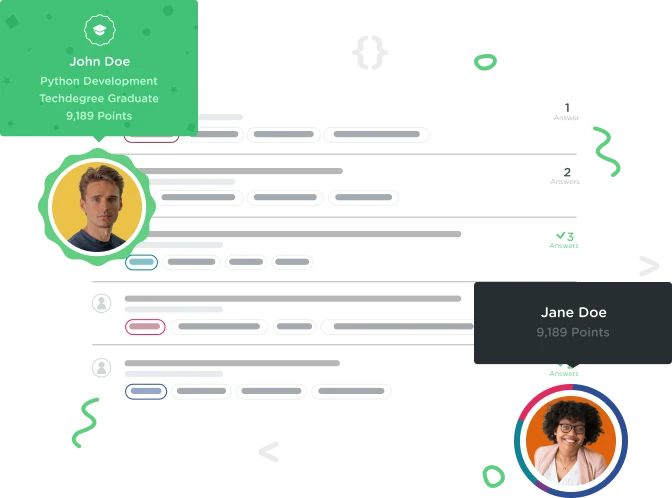

Geoff Ruddock
2,573 PointsRemoving non-ints from a list
Trying to remove non-ints using the code below, but getting an unexpected result.
Code:
the_list = ["a", 2, 3, 1, False, [1, 2, 3]] for item in the_list: if type(item) is not int: the_list.remove(item) print(the_list)
Gives me the result: [2, 3, 1, [1, 2, 3]]
Does anyone know why this happens? Although I can get around it for the quiz question, I am curious why this approach is failing.
2 Answers

Fable Turas
9,405 PointsThe reason you cannot remove items in a list while iterating over it is because once you remove an item the index of all the remaining items changes and so the item immediately following the removed item will be skipped when the code loops around for the next iteration.
For example, at the start of your code "a" is at index 0, and 2 is at index 1, but as soon as "a" is removed the index point for 2 becomes 0. But the for loop doesn't know this and only knows to move on to the next item, which is now 3 and 2 is never evaluated. This becomes more important when False is removed and [1, 2, 3] gets bumped up an index.
There is, however, another way around this aside from creating 2 lists, and that is to iterate through the list backwards.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
for item in reversed(the_list):
if type(item) is not int:
the_list.remove(item)
print(the_list)

Chris Bennett
7,499 PointsSo in Python you can't remove items from a list while you are iterating over it.
(you technically can but you get funky results)
A simple solution would be to create a temporary list and append all the ints to that list then make the_list = temp_list. There's shorthand to do this by the way but I think this code is easier to understand.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
temp_list = []
for item in the_list:
if type(item) == int:
temp_list.append(item)
the_list = temp_list
print the_list

Geoff Ruddock
2,573 PointsThanks for your help. This is a very clear and logical way to solve it.
Geoff Ruddock
2,573 PointsGeoff Ruddock
2,573 PointsVery clever! Thanks for this workaround!