Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial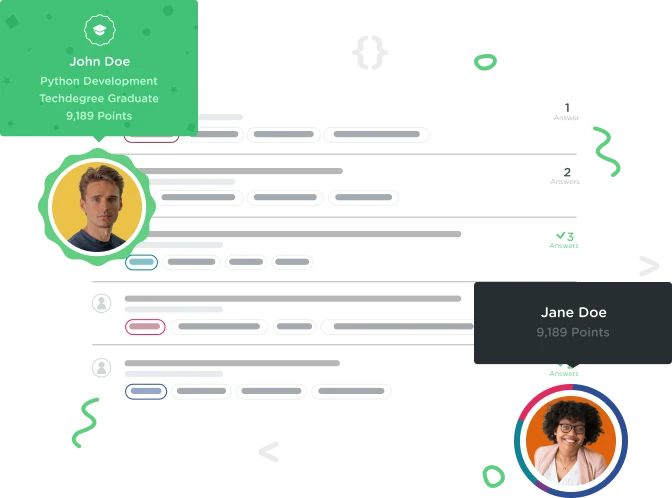

Guilherme Kunzler
1,433 PointsRemoving "self"
I've removed all "self"s from my code and it still works.
We use self for best practice? Or there is something I didn't learn in the course yet that will make self more useful?
*************************** CODE ************************
struct Contact {
let firstName: String
let lastName: String
var type: String
init(fName: String, lName: String){
firstName = fName
lastName = lName
type = "Friend"
// self.firstName = fName // self.lastName = lName // self.type = "Friend"
}
func fullName() -> String {
return firstName + " " + lastName
}
func findType ()-> String{
return type
}
}
var person = Contact(fName: "gui", lName: "maia") person.fullName() person.findType()
2 Answers
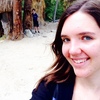
Lauren Hibbs
1,872 PointsSelf is useful mainly when and object is passing a reference of itself to another class, function, etc. It isn't necessary within a class because it already has access to all of its properties and functions.
struct School {
var name : String
var students: [Student] = []
init(schoolName : String){
students = []
name=schoolName
}
mutating func addStudent(student : Student){
students.append(student)
}
}
struct Student{
var name :String
var aSchool :School
mutating func enroll(){
aSchool.addStudent(self)
}
}
var mySchool = School(schoolName:"Random School")
var person = Student(name:"Lauren", aSchool:mySchool)
person.enroll()
My experience is in Java, not Swift, so this might be a little complicated but hopefully it is right. Hope this helps!

Ryan Ebbers
3,746 PointsFrom my understanding of the keyword "self", it explicitly says that you are targeting the property of a specific Struct instance. Using Amit's Car example, "self" declares that you are working with this Car instance and not one of the other instances.
On a side note, I started to remove "self" from my playground to see if it still worked on my computer. It still worked, but only after modifying the init() method parameter names (I was using "firstName" and "lastName", and changed them to "fName" and "lName"). As Amit said in the previous course, it is good practice to use descriptive parameter names.
In closing, using the "self" keyword targets that exact instance and allows you to use the same variable and parameter name (when you prepend "self" to the variable name) within the init() method.
Hope this helps.