Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial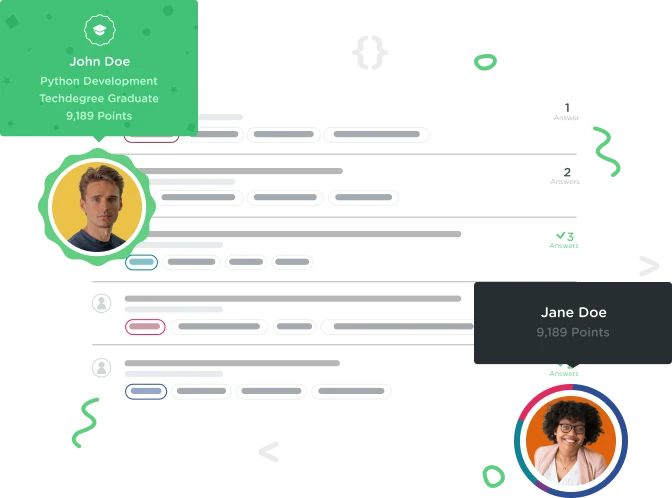

S Campos
413 PointsRemoving the last div within a div
I was trying to play with the code on my own -- trying to delete div childs within another div. Tried using :last-child but was having a hard time finally found:last-of-type I have two questions:
Question #1) does :last-child work on classes? I couldn't get it to work. And didnt see any examples on mdn using it on classes just on types like <p> and <li> but never on divs or classes and ID's.
Question #2)
In the example, his
document.querySelector('li:last-child');
the li:last-child has no space in between li and :
In the following code on line 4:
const div10RemoeButtonVariable = document.getElementById('div10removeButton');
div10removeButton.addEventListener('click', () => {
let lastBoxVariable = document.querySelector(".boxes :last-of-type")
divBoxVar.removeChild(lastBoxVariable);
});
why does
.boxes :last-of-type
work
and
.boxes:last-of-type
not work
ie the space.
Here's my code
HTML
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
<meta charset="utf-8"/>
</head>
<body>
<div class="div9">
<h1> Adding elements</h1>
<button id="button9">Add boxes </button>
<br>
<div class="boxes">
<div class="red-box"></div>
</div> <!-- boxes end -->
</div><!-- div9end -->
<div class='10'>
<h1>Removing elements</h1>
<button id="div10removeButton">remove boxes</button>
</div><!-- div10end -->
<script src="app.js"></script>
</body>
</html>
CSS
.boxes{
display:inline-flex;
}
.red-box{
width:50px;
height:30px;
background-color: red;
margin: 5px;
}
Javascript
//example9
const button9Var = document.querySelector('#button9');
const divBoxVar = document.querySelector(".boxes");
button9.addEventListener('click', ()=>{
let newBoxVariable = document.createElement('div');
newBoxVariable.className = 'red-box';
divBoxVar.appendChild(newBoxVariable);
});
//example10
const div10RemoeButtonVariable = document.getElementById('div10removeButton');
div10removeButton.addEventListener('click', () => {
let lastBoxVariable = document.querySelector(".boxes :last-of-type")
divBoxVar.removeChild(lastBoxVariable);
});
Sorry it's a lot! Hopefully someone out there knows the answers I seek!
2 Answers
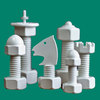
Steven Parker
231,269 PointsAny pseudo class, like ":last-of-type
", can be combined with other selectors using tag names, classes, id's, etc. But be aware that the designation "last of type" refers to within the item's parent element, even if that parent element is not specified in the selector.
In the HTML code shown above, the red box is both the last child and the last of type, so either pseudo-selector should target it.
And a space in a selector indicates a descendant relationship. so ".boxes :last-of-type
" means "an item which is the last of its type that is inside another element with class boxes", but ".boxes:last-of-type
" means "an item which is the last of its type that has the class boxes". The first example would target the red box, but the second one would target the div
that has the class "boxes" instead (since it is also a last of type).

S Campos
413 PointsWOW! Steven Parker Thank you so much for your in depth explanation!! I greatly appreciate it