Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial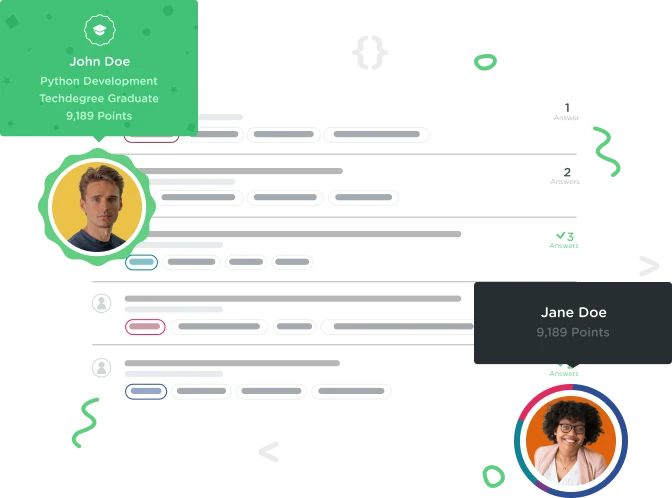

Therman Trotman
Courses Plus Student 376 PointsRender url in Datatables
Hey Everyone,
I am using the jQuery plugin, Datatables. I'm displaying data from a SharePoint list in a datatable using this code:
$(document).ready(function() {
var RestUrl = "../_api/Web/Lists/GetByTitle('BrandItems')/items";
$.ajax({
url: RestUrl,
method: "GET",
headers: {
"accept": "application/json;odata=verbose",
},
success: function(data) {
if (data.d.results.length > 0) {
//construct HTML Table from the JSON Data
$('#DatatableGrid').append(GenerateTableFromJson(data.d.results));
//Bind the HTML data with Jquery DataTable
var oTable = $('#EmployeeTable').dataTable({
"iDisplayLength": 10,
"aLengthMenu": [
[5, 10, 30, 50],
[5, 10, 30, 50]
],
"sPaginationType": "full_numbers"
});
} else {
$('#DatatableGrid').append("<span>No Employee Details Found.</span>");
}
},
error: function(data) {
$('#DatatableGrid').append("<span>Error Retreiving Employee Details. Error : " + JSON.stringify(data) + "</span>");
}
});
function GenerateTableFromJson(objArray) {
var tableContent = '<table id="EmployeeTable" style="width:100%"><thead><tr><td>File Name</td>' + '<td>File Type</td>' + '<td>Organization</td>' + '<td>Download</td>' + '</tr></thead><tbody>';
for (var i = 0; i < objArray.length; i++) {
tableContent += '<tr>';
tableContent += '<td>' + objArray[i].Display_x0020_Name + '</td>';
tableContent += '<td>' + objArray[i].FileType0 + '</td>';
tableContent += '<td>' + objArray[i].Organization + '</td>';
tableContent += '<td>' + objArray[i].DL_Link + '</td>';
tableContent += '</tr>';
}
return tableContent;
}
});
The "tableContent += '<td>' + objArray[i].DL_Link + '</td>';" line shows [object Object] when rendered in the datatables. It's a URL field. After an intense amount of Googling, I came across this piece of example code:
{
"mData": "ID",
"render": function(mData, type, row, meta) {
var returnText = "";
var url = _spPageContextInfo.webAbsoluteUrl + "/Lists/ProjectDetails/DispForm.aspx?ID=" + mData;
returnText = "<a target='_blank' href=" + url + ">" + mData + "</a>";
return returnText;
}
}
But I don't grasp the concept of how to add it to my existing code in order to display the url column.
Can someone explain how I would do that?
Appreciate any help.
1 Answer
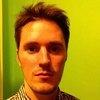
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsCan you print objArray[i].DL_Link
to the console, what does it look like? Maybe you need to access some property inside of it.
Also, template literals would be very handy here to build up these sections of HTML, rather than using concatenation.