Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial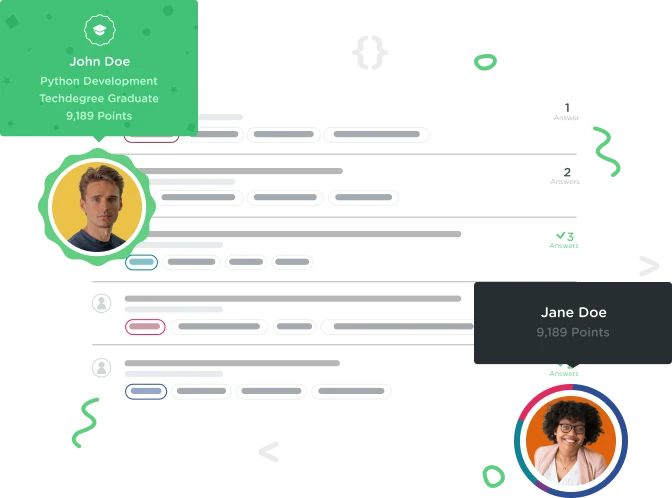
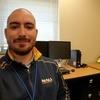
Ryan Morales
Full Stack JavaScript Techdegree Graduate 33,933 PointsRendering react .map components after filtering the returned objects.
If I create two separate react components, how do I integrate them upon rendering? For example, I want to create a list of science topics and a separate list of science videos. When I return each component as an object {topics} and {videos} they will be listed out as all of the topics and then all of the videos. How can I filter them by props and then write them out as topic1, video1, topic2, video2 etc.
1 Answer

Robert Adamson
5,741 PointsYou need a parent component that maintains the state (your topics) and loops through them to render topic headers and video collections related to each one. This is called 'lifting state'. I'm assuming you want to filter videos by topic. You need to iterate through topics in the parent component, then render your 'topic' component passing the topic down to it, and render the video collection component that returns videos filtered by this topic. Here is a rough untested example to give you an idea:
// Parent component where the shared state lives
// This loops through all your topics and renders a topic headline and a video collection of the related topic
const ParentComponent = () => {
// Your shared state
const topics = ['topic 1', 'topic 2', 'topic 3'];
// Loops through your topics and returns the correct topic headline and video collection
const generateVideos = () => {
topics.map(topic => {
return (
<div className="topicContainer">
<TopicComponent topic={topic} />
<VideoCollectionComponent topic={topic} />
</div>
);
});
};
// Renders this component and all of the videos you wanted to show/filter
return (
<div className="parentContainer">
{generateVideos}
</div>
);
};
// Component rendering your topic header
const TopicComponent = (props) => {
return (
<div className="topicContainer">
<h2>{props.topic}</h2>
</div>
);
};
// Component rendering your video collections
const VideoCollectionComponent = (props) => {
// Your video data
const videoList = [
{uri: 'http://path/to/video', topic: 'topic 1'},
{uri: 'http://path/to/video', topic: 'topic 2'},
{uri: 'http://path/to/video', topic: 'topic 3'},
];
// Filters all of your videos down to just the ones in this topic
const filterVideosByTopic = (topic) => {
return videoList.filter(video => {
return video.topic === topic;
});
}
// Takes all of the videos you filtered down and generates some video component that might live somewhere in your codebase
const generateVideoPlayers = () => {
const videosToRender = filterVideosByTopic(props.topic);
return videosToRender.map(video => {
return (
<VideoPlayerComponent uri={video.uri} />
);
});
};
return (
<div className="videoContainer">
{generateVideoPlayers}
</div>
);
};
Recommended reading
Lifting state: https://reactjs.org/docs/lifting-state-up.html