Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial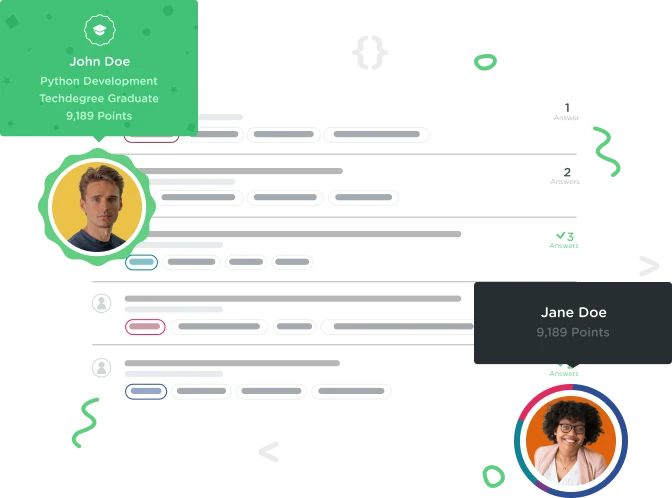
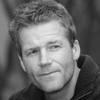
Maarten De Kruif
7,314 PointsRepeatdetector
Not All code paths return a value?
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector:SequenceDetector
{
public override bool Scan(int[] sequence)
{
int previous = 0;
for(int i=1;i<=sequence.Length;i++)
{
int current=sequence[i];
if(previous==current)
{
return true;
}
previous=sequence[i];
}
}
}
}
2 Answers
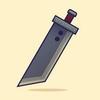
Allan Clark
10,810 PointsYour only return statement is enclosed in an if statement, so should the if statement be skipped bc it evaluates to false, there is no return statement for the method to execute. Adding 'return false;' just after you're for loop should work. this will guarantee that if it goes all the way through the loop without returning, it will always return false.
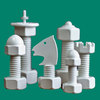
Steven Parker
231,268 PointsAnytime you return a value inside a conditional, you should also do so after the conditional.
In this case it would also be after the loop. The compiler can tell that if the condition never passes, the method will also not return a bool value as promised by the declaration. So the last statement of the method should be "return false;
".